Agile, test-driven development
It All Started with a Test
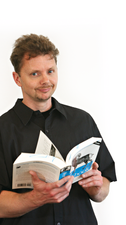
Test-driven development with a full-coverage regression test suite as a useful side effect promises code with fewer errors. Mike "Perlmeister" Schilli enters the same path of agility and encounters a really useful new CPAN module.
A few weeks ago, my employer sent me to a course about test-driven development (TDD) and agile methods, and to implement my newly gained knowledge practically, I am dedicating today's Perl snapshot to this principle.
Fast and flexible developers typically set off immediately without paying attention to details. They always write a test first before they get down to implementing a function. The test suite thus grows automatically with tests relevant to system functions. They clean up dirty code by refactoring later; this is possible without any risk thanks to the safety net provided by the test suite.
Nothing but Errors – and Rightly So
The tests developed before writing a function will fail, of course, because the desired feature either does not exist or is only partially or incorrectly implemented at first. When the code arrives later on, the test suite passes and turns to green; development environments such as Eclipse actually visualize it this way.
[...]