Strange Coincidence
Programming Snapshot – Python Gambling
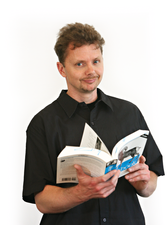
Can 10 heads in a row really occur in a coin toss? Or, can the lucky numbers in the lottery be 1, 2, 3, 4, 5, 6? We investigate the law of large numbers.
If you follow the stock market, you are likely to hear people boasting that their buy and sell strategies allegedly only generate profits and never losses. Anyone who has read A Random Walk down Wall Street [1] knows that wild stock market speculation only leads to player bankruptcy in the long term. But what about the few fund managers who have managed their deposits for 20 years in such a way that they actually score profits year after year?
According to A Random Walk down Wall Street, even in a coin toss competition with enough players, there would be a handful of "masterly" throwers who, to the sheer amazement of all other players, seemingly throw heads 20 times in a row without tails ever showing up. But how likely is that in reality?
Heads or Tails?
It is easy to calculate that the probability of the same side of the coin turning up twice in succession is 0.5x0.5 (i.e., 0.25), since the probability of a specific side showing once is 0.5 and the likelihood of two independent events coinciding works out to be the multiplication of the two individual probabilities. The odds on a hat trick (three successes in a row) are therefore 0.5^3=0.125, and a series of 20 is quite unlikely at 0.5^20=0.000000954. But still, if a program only tries often enough, it will happen sometime, and this is what I am testing for today. The short Python coin_throw()
generator in Listing 1 [2] simulates coin tosses with a 50 percent probability of heads or tails coming up.
[...]