Testing the Adafruit PyPortal touchscreen
Display Deluxe
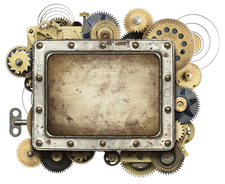
© Lead Image © donatos1205, 123RF.com
Unlike other displays for the Raspberry Pi, Adafruit's PyPortal touchscreen provides an autonomous environment, including a microprocessor, sound output, and a WiFi connection.
The Raspberry Pi has a hard time with small displays because support from Raspbian is surprisingly poor. Creating and implementing suitable interfaces is difficult with a dearth of off-the-shelf programs for controlling small displays. I'm quietly confident that the PyPortal intelligent touchscreen by Adafruit is a better solution.
PyPortal (Figure 1) is a small 3.2-inch networkable resistive touch display with an integrated microprocessor [1]. At $55 (EUR59), it is not exactly cheap, but considering its components, the price seems reasonable. A correspondingly sized 3.2-inch display plus a Pi Zero W with an SD card, at about the same price, will serve as a comparison with the PyPortal configuration.
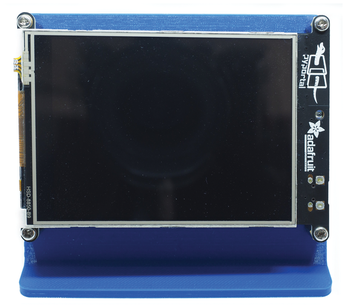
[...]