Search for processes by start time
Ghost Hunter
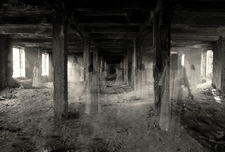
© Lead Image © batareykin, 123RF.com
How do you find a process running on a Linux system by start time? The question sounds trivial, but the answer is trickier than it first appears.
As the maintainer of a computing cluster [1], Frank also provides his users with commercial software for calculations based on the fair-use principle. A limited number of license keys are available for this software (e.g., 10 keys for the MATLAB [2] simulation software).
Some of these calculations can take up to a week. When a calculation is finished and the process terminates, the license key is automatically returned to the pool of free keys and can be grabbed by another user. However, if users forget to end their processes, no more keys can be handed out, as these have all been allocated. To prevent this, the admins want to automatically search for processes that are older than 10 days. If they find a process matching this criteria, they can check with the users to clarify what should happen to the process.
The Linux kernel manages processes and makes information relating to them available to the user in the /proc
filesystem. At the command line, ps
is the reliable interface to process management. Unfortunately, ps
has dozens of options, and its output is often not very clear either. This can be remedied with a little shell code or possibly a scripting language. This article compares several potential solutions using Bash, Python and Perl scripts, and the Go programming language.
[...]