Simple DirectMedia Layer 2.0
Newcomer-Friendly
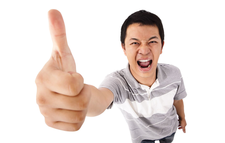
© Lead Image © tomwang, 123RF.com
After several years of development work, version 2.0 of the SDL library was released in August 2013. Despite its many innovations, migrating to and getting started with SDL 2.0 is amazingly easy.
Since 1998, Simple DirectMedia Layer (SDL) has formed the basis for countless games, multimedia applications, and even virtualization. The small cross-platform library makes it easy for applications to access the graphics card, the audio hardware, the keyboard, and other input devices. Because it is available for many different platforms and operating systems, you can easily port any programs that have been developed with it (see the "Supported Operating Systems" box). Without SDL, Linux users would probably still be waiting for conversions of blockbuster games like Portal (Figures 1 and 2).
For an amazing 12 years, SDL version 1.2 provided the underpinnings of numerous programs. Changes have only come in small doses, with the last revision (1.2.15) appearing in January 2012. SDL 1.2 is thus considered extremely robust, but hardware development has mercilessly left it behind. In the background, SDL developers have already been working for several years in parallel on a revamped, modern variant.
The developer versions initially had a version number of 1.3, but – because of the many internal changes – the developers made the leap to 2.0 in February 2012. Although this new branch had already been used in many software projects, the developers were reluctant to put a "Stable" stamp on it. In August 2013, however, SDL inventor and project manager Sam Lantinga drew the line and officially released the current state of development as version 2.0.0.
[...]