Scraping highly dynamic websites
Programming Snapshot – chromedp
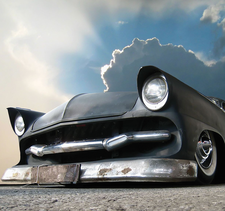
© Lead Image © Rene Walter, 123RF
Screen scrapers often fail when confronted with complex web pages. To keep his scraper on task, Mike Schilli remotely controls the Chrome browser using the DevTools protocol to extract data, even from highly dynamic web pages.
Gone are the days when hobbyists could simply download websites quickly with a curl
command in order to machine-process their content. The problem is that state-of-the-art websites are teeming with reactive design and dynamic content that only appears when a bona fide, JavaScript-enabled web browser points to it.
For example, if you wanted to write a screen scraper for Gmail, you wouldn't even get through the login process with your script. In fact, even a scraping framework like Colly [1] would fail here, because it does not support JavaScript and does not know the browser's DOM (Document Object Model), upon which the web flow relies. One elegant workaround is for the scraper program to navigate a real browser to the desired web page and to inquire later about the content currently displayed.
For years, developers have been using the Java Selenium suite for fully automated unit tests for Web user interfaces (UIs). The tool speaks the Selenium protocol, which is supported by all standard browsers, to get things moving. Google's Chrome browser additionally implements the DevTools protocol [2], which does similar things, and the chromedp project on GitHub [3] defines a Go library based on it. Go enthusiasts can now write their unit tests and scraper programs natively in their favorite language. I'll take a look at some screen-scraping techniques in this article, but keep in mind that many websites have licenses that prohibit screen scraping. See the site's permission page and consult the applicable laws for your jurisdiction.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
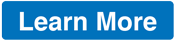
News
-
There's a New Linux AI Assistant in Town
Newelle is a Linux AI assistant that can work with different LLMs and includes document parsing and profiles.
-
Linux Kernel 6.16 Released with Minor Fixes
The latest Linux kernel doesn't really include any big-ticket features, just a lot of lines of code.
-
EU Sovereign Tech Fund Gains Traction
OpenForum Europe recently released a report regarding a sovereign tech fund with backing from several significant entities.
-
FreeBSD Promises a Full Desktop Installer
FreeBSD has lacked an option to include a full desktop environment during installation.
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.