Organizing photos by date with Go
Marking the Cards
In this way, Read()
from line 54 reads the data from the cache file and turns it into a Go map that assigns photo paths to Boolean values. To do this, it uses os.Open()
to open the file and calls in a scanner from the bufio package for a new scanner starting in line 62. It calls Scan()
in line 63 to browse through every single line of the cache file and then calls Text()
to fetch the matching text as a string, excluding the line break.
The assignment in line 65 creates a key in the cache
map for each cache entry and assigns a value of true
to it. The cache.cache
map remains stored in the instance structure, where other functions like cache.Exists()
or cache.Set()
can access it later.
To update the cache file after completing the work, the Write()
function starting in line 71 writes the modified map back. To do this, it calls OpenFile()
to open the cache file in line 72 and iterates over the map entries to write them back to the cache file one by one with fmt.Fprintf
, overwriting the old ones because of the O_TRUNC
option.
Previously unseen SD cards do not have .uuid
files in their root directories. The Init()
function starting in line 34 checks for this and creates a new UUID with the uuid GitHub package from Google in line 40 if line 39 failed to find one previously. This 36-character string is guaranteed to be unique each time, so it will continue to uniquely identify cards marked with it in the future [2].
Date from Exif Headers
The date a photo was taken is determined by the function photoDate()
starting in line 11 in Listing 2. The exif package from the goexif2 project on GitHub provides convenient functions that read the Exif header of a JPEG image, decode it, and return it as a Go time.Time
type variable. Its functions Year()
, Month()
, and Day()
convert the photo date into year, month, and day. importer
relies on this later on to create the nested file structure for keeping the photos organized in storage.
Listing 2
util.go
01 package main 02 03 import ( 04 "fmt" 05 exif "github.com/xor-gate/goexif2/exif" 06 "io" 07 "os" 08 "path" 09 ) 10 11 func photoDate(path string) ([]int, error) { 12 dt := []int{} 13 14 f, err := os.Open(path) 15 if err != nil { 16 return dt, err 17 } 18 19 x, err := exif.Decode(f) 20 if err != nil { 21 return dt, err 22 } 23 24 t, err := x.DateTime() 25 if err != nil { 26 return dt, err 27 } 28 29 return []int{int(t.Year()), int(t.Month()), int(t.Day()), 30 int(t.Hour()), int(t.Minute()), int(t.Second())}, nil 31 } 32 33 func copy(src, dst string) (int64, error) { 34 sourceFileStat, err := os.Stat(src) 35 if err != nil { 36 return 0, err 37 } 38 39 if !sourceFileStat.Mode().IsRegular() { 40 return 0, fmt.Errorf("%s is not a regular file", src) 41 } 42 43 source, err := os.Open(src) 44 if err != nil { 45 return 0, err 46 } 47 defer source.Close() 48 49 dest, err := os.Create(dst) 50 if err != nil { 51 return 0, err 52 } 53 defer dest.Close() 54 nBytes, err := io.Copy(dest, source) 55 return nBytes, err 56 } 57 58 func targetDir() string { 59 homedir, err := os.UserHomeDir() 60 panicOnErr(err) 61 return path.Join(homedir, "/idb") 62 }
However, the Go standard library does not have a function for copying files. This is why copy()
has to open the source and target files starting in line 33, read them block by block from the source
, and write them to the target dest
with io.Copy()
. The archive directory for the importer is idb/
in the user's home directory; the path is determined and returned by the targetDir()
function starting in line 58 of Listing 2.
In the main program in Listing 3, main()
first checks whether the call also includes a directory for importing photos. After reading the cache file in line 35, the Walk()
function from the standard filepath package plumbs the depths of the specified import directory and processes all the JPEG files it finds there.
Listing 3
importer.go
01 package main 02 03 import ( 04 "errors" 05 "flag" 06 "fmt" 07 "os" 08 "path" 09 "path/filepath" 10 rex "regexp" 11 ) 12 13 func main() { 14 flag.Usage = func() { 15 fmt.Printf("Usage: %s dir\n", path.Base(os.Args[0])) 16 os.Exit(1) 17 } 18 19 flag.Parse() 20 if flag.NArg() < 1 { 21 flag.Usage() 22 } 23 24 idir := flag.Args()[0] 25 26 tDir := targetDir() 27 _, err := os.Stat(tDir) 28 if errors.Is(err, os.ErrNotExist) { 29 err := os.Mkdir(tDir, 0755) 30 panicOnErr(err) 31 } 32 33 cache := NewCache(idir) 34 cache.Init() 35 cache.Read() 36 37 filepath.Walk(idir, func(ipath string, f os.FileInfo, err error) error { 38 jpgMatch := rex.MustCompile(`(?i)^\w.*JPG$`) 39 dir, bpath := path.Split(ipath) 40 match := jpgMatch.MatchString(bpath) 41 if !match { 42 return nil 43 } 44 45 dir = path.Base(dir) 46 twoPath := path.Join(dir, bpath) // parent/file 47 48 ok := cache.Exists(twoPath) 49 if ok { 50 return nil // already archived 51 } 52 53 dt, err := photoDate(ipath) 54 if err != nil { 55 fmt.Printf("Error: %s: %s\n", ipath, err) 56 return nil 57 } 58 dstDir := fmt.Sprintf("%s/%d/%02d/%02d", tDir, dt[0], dt[1], dt[2]) 59 os.MkdirAll(dstDir, 0755) 60 newFile := path.Base(ipath) 61 dst := fmt.Sprintf("%s/%d%02d%02d%02d%02d%02d-%s", 62 dstDir, dt[0], dt[1], dt[2], dt[3], dt[4], dt[5], newFile) 63 fmt.Printf("Copying %s to %s\n", ipath, dst) 64 _, err = copy(ipath, dst) 65 panicOnErr(err) 66 67 cache.Set(twoPath) 68 return nil 69 }) 70 71 cache.Write() 72 } 73 74 func panicOnErr(err error) { 75 if err != nil { 76 panic(err) 77 } 78 }
JPEGs Only
The regular expression in line 38 of Listing 3 filters out all non-JPEGs and tells the walker to return without touching any foreign objects. If the file is obviously a regular photo, then line 39 breaks down the path into the parent directory and file name, while line 45 truncates everything but the last subpath from the directory. Based on this and the file name, line 46 in twoPath
then creates the short path consisting of the parent directory and file name that the cache uses as a key later.
Line 48 checks if the short path already exists in the cache (i.e., if the file has been archived before). If so, the Walk()
callback returns in line 50 without taking any further action. But if a previously unarchived photo is found, photoDate()
in line 53 extracts the year, month, and day the photo was taken from the photo's Exif header. From this, the function determines the target directory in the archive, as idb/<Year>/<Month>/<Day>
, and then creates it if it does not already exist.
Now it's time to copy the photo to the archive. Line 61 once again adds the date the photo was taken to the name of the target file in the archive directory. The reason for this apparent redundancy is the idb
tool from the last issue [2], which uses the -xlink
option to link all tagged photos to a directory. If we only used the original file name for archived photos, several photo files with the name DSC00001.JPG
could end up in the search results because the consecutive numbers are used again and again by the camera on reformatted cards.
After completing the copying work, line 67 records the file and the card's UUID in the cache; line 71 writes the cache back to the disk at the end of the function.
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
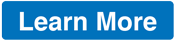
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.