Automated Photo Sharing with Photocrumbs
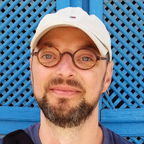
Productivity Sauce
Using a simple Bash script and a cron job, you can turn Photocrumbs into an automated photo sharing bot. This way, you can drop multiple photos into a separate directory, and the script/cron job combo will publish them one-by-one at specified intervals (e.g., hourly, daily, etc.). The basic version of the Bash script is simplicity itself:
#!/bin/bash FILE=$(ls *.{jpeg,jpg,JPG,JPEG} | head -1) mv $FILE /var/www/photocrumbs/photos/
The script uses the ls and head tools to obtain the name of the first photo in the current directory. The script then copies the photo to the photos directory inside the Photocrumbs installation. To set up the script, create a text file and paste the code above into it. Save the script under the photobot.sh name, and make it executable using the chmod +x photobot.sh command. Next, you need to create a cron job that will run the script at a specified schedule. Use the crontab -e command to open the crontab file for editing and add the following job to it (replace path/to/dir with the actual path to the directory containing the script and the photos):
daily cd path/to/dir ./photobot.sh
This job will run the script once a day, but you can specify any other schedule. Of course, this basic script can be improved in a number of ways. For example, you can add a condition which aborts the script if there are no photos in the directory:
#!/bin/bash FILE=$(ls *.{jpeg,jpg,JPG,JPEG} | head -1) if [[ -n "$FILE" ]]; then mv $FILE /var/www/photocrumbs/photos/ else exit 0 fi
Since Photocrumbs can pull descriptions from accompanying .php files, you can tweak the script to generate a .php file with predefined text for each photo.
#!/bin/bash FILE=$(ls * | head -1) TEXT="Monkey loves banana" if [[ -n "$FILE" ]]; then PHP_FILE="${FILE%%.*}".php echo $TEXT > $PHP_FILE mv $FILE /var/www/photocrumbs/photos/ mv $PHP_FILE /var/www/photocrumbs/photos/ else exit 0 fi
Another variation of the script grabs a random line from the text.txt file and uses the obtained text as a description of the published photo:
#!/bin/bash FILE=$(ls * | head -1) if [[ -n "$FILE" ]]; then TEXT=$(shuf -n 1 text.txt) PHP_FILE="${FILE%%.*}".php echo $TEXT > $PHP_FILE mv $FILE /var/www/photocrumbs/photos/ mv $PHP_FILE /var/www/photocrumbs/photos/ else exit 0 fi
Of course, this technique is not limited to Photocrumbs: you can easily adapt the script to work with any other photo sharing application that publishes photos by pulling them from a specific directory.
comments powered by DisqusSubscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
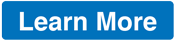
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.