Back up MySQL Databases with a Simple Bash Script
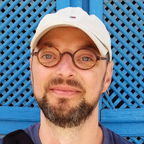
Productivity Sauce
If you host your own blog or any Web-based application running on the Apache/MySQL/PHP stack, you should have a backup system in place for keeping data stored in MySQL databases safe. There are several solutions that can help you with that, but nothing beats a simple Bash script I stumbled upon in a blog post comment. Here is the script in all its beauty:
#!/bin/bash NOW=`date +"%Y-%m"`; BACKUPDIR="location/of/your/backup/dir/$NOW"; ### Server Setup ### #* MySQL login user name *# MUSER="user"; #* MySQL login PASSWORD name *# MPASS="pass"; #* MySQL login HOST name *# MHOST="your-mysql-ip"; MPORT="your-mysql-port"; # DO NOT BACKUP these databases IGNOREDB=" information_schema mysql test " #* MySQL binaries *# MYSQL=`which mysql`; MYSQLDUMP=`which mysqldump`; GZIP=`which gzip`; # assuming that /nas is mounted via /etc/fstab if [ ! -d $BACKUPDIR ]; then mkdir -p $BACKUPDIR else : fi # get all database listing DBS="$(mysql -u $MUSER -p$MPASS -h $MHOST -P $MPORT -Bse 'show databases')" # SET DATE AND TIME FOR THE FILE NOW=`date +"d%dh%Hm%Ms%S"`; # day-hour-minute-sec format # start to dump database one by one for db in $DBS do DUMP="yes"; if [ "$IGNOREDB" != "" ]; then for i in $IGNOREDB # Store all value of $IGNOREDB ON i do if [ "$db" == "$i" ]; then # If result of $DBS(db) is equal to $IGNOREDB(i) then DUMP="NO"; # SET value of DUMP to "no" #echo "$i database is being ignored!"; fi done fi if [ "$DUMP" == "yes" ]; then # If value of DUMP is "yes" then backup database FILE="$BACKUPDIR/$NOW-$db.gz"; echo "BACKING UP $db"; $MYSQLDUMP --add-drop-database --opt --lock-all-tables -u $MUSER -p$MPASS -h $MHOST -P $MPORT $db | gzip > $FILE fi done
The best part is that you only need to specify a handful of parameters to make the script work. This includes BACKUPDIR (the destination for storing backups), MUSER (MySQL user), MPASS (MySQL user password), MHOST (the IP address of the MySQL server, e.g. localhost), and MPORT (the port the MySQL database is running on, default is 3306).
You can run the script manually, or you can set up a cron job which will perform backups on a regular basis. To do this, run the crontab -e command and add the following line (replace the sample path with the actual path and backup script name):
@daily /path/to/mysqlbackupscript.sh
Don't forget to make the script executable using the chmod a+x mysqlbackupscript.sh command.
Comments
comments powered by DisqusSubscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
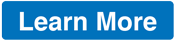
News
-
There's a New Linux AI Assistant in Town
Newelle is a Linux AI assistant that can work with different LLMs and includes document parsing and profiles.
-
Linux Kernel 6.16 Released with Minor Fixes
The latest Linux kernel doesn't really include any big-ticket features, just a lot of lines of code.
-
EU Sovereign Tech Fund Gains Traction
OpenForum Europe recently released a report regarding a sovereign tech fund with backing from several significant entities.
-
FreeBSD Promises a Full Desktop Installer
FreeBSD has lacked an option to include a full desktop environment during installation.
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
simple & compact version
NOW=`date +%Y-%m-%d`;
BKPDIR=/path/to/backup/$NOW;
mkdir -p $BKPDIR;
for DB in $(echo 'SHOW DATABASES;' | mysql -uuser -ppassword | grep -v '^Database$'); do
if [[ "${IGNOREDB}" =~ "#${DB}#" ]]; then continue; fi
mysqldump -uuser -ppassword --opt --add-drop-database --lock-all-tables --max_allowed_packet=500M $DB | bzip2 > $BKPDIR/$NOW-$DB.sql.bz2;
done;
Oh the horror
A shorter script using regular expression support
# DO NOT BACKUP these databases
IGNOREDB="#information_schema#mysql#test#"
...
for db in $DBS
do
if [ "${IGNOREDB}" =~ "#${db}#" ]; then continue; fi
FILE="$BACKUPDIR/$NOW-$db.gz";
echo "BACKING UP $db";
$MYSQLDUMP --add-drop-database --opt --lock-all-tables -u $MUSER -p$MPASS -h $MHOST -P $MPORT $db | gzip > $FILE
done
Avoiding the second inner loop and the $DUMP variable.
My two cents!
Backing up large databases
You'll want to add something like --max_allowed_packet=500M to the mysqldump command line to work around that.