Write standards-compliant C# programs in Linux with DotGNU
Working with the Compiler
For those who speak binary, DotGNU works by transforming bytecode into a simple instruction set that is passed on to a virtual machine to be executed via an interpreter. This design makes DotGNU easily portable and explains the number of supported platforms.
The components you'll use are the ilrun run-time engine, which executes the binaries doled out via the cscc compiler, and cscc some-program.cs, which produces a file called a.out. This file can then be executed with:
ilrun a.out
With the -o switch, you can specify a file name when compiling your program with cscc – for instance:
cscc -o some-program.exe some-program.cs
To print debugging information, use the -v switch while compiling.
If you have to link against a particular library, say System.Drawing, you'll need to point this out to the compiler with the -lLIBRARY switch, which will search for the libraries DLL along the library search path. If you are compiling a GUI program that uses the System.Windows.Forms library, you can also use the -winforms switch, which automatically links all the libraries required to process a WinForms-dependent program. Sometimes you'll have to create your own DLL libraries. The -shared switch will produce these DLLs instead of a .exe.
In addition to C#, the DotGNU compiler can also compile to Java Virtual Machine bytecode with the -mjvm switch. Remember to use the .jar file extension instead of .exe or .dll.
Dive In
After that brief introduction to the compiler and common options, I'll honor the long tradition of coding tutorials by writing a "Hello, World" program in C# (Listing 1) and compiling it with cscc -o hello.exe hello.cs.
Listing 1
Hello.cs
The simple program contains only one method: Main(). Command-line arguments are passed to this method as an array of string objects by way of the System library and its various classes and methods. System.Environment.Exit() exits the program and sends a return code to the shell. The System.Console class interfaces the command line to the program. The Console.Writeline() method writes the greeting to standard output, and Console.Error.Writeline() writes to standard error.
Listing 1 greets the user with a name slipped in as input. But why enter a name for the user when a name is probably already defined on the system? Using the System.Collections library, you can, among other things, display the contents of all the environment variables. If you modify the hello.cs program to use the System.Collections library (Listing 2), you can read the username of the user executing the program with value = Environment.GetEnvironmentVariable("USER"), wherein value is a String type variable.
Listing 2
Hello-Advanced.cs
Get Set GUI
One of the best bits about DotGNU is an implementation of the System.Windows.Forms library that doesn't require translation via other popular toolkits such as Gtk. Much like the Java Swing library, DotGNU's System.Windows.Forms draws its own controls.
To compile the code in Listing 3, use:
cscc -o form.exe form.cs -winforms.
The code displays a simple re-sizable window with the usual minimize, maximize, and close controls.
Listing 3
Using System.Windows.Forms
The next step is to add a menu to this window. The complete listing is available on the magazine website [2], but the most important bits are in Listing 4. A custom class called MyMenu inherits from the System.Windows.Forms.MainMenu class and is used to create the menu items. The menu item variables are of the MenuItem type, which is specifically used to create items within a menu or a context menu.
Listing 4
Advanced Form Controls
The MyMenu() constructor creates the File menu item with the new keyword. Similarly, Listing 4 creates an instance of the three menu items and specifies how they'll appear in the menu. Because I already have the File menu ready, I use the Add() method to add the three menu items to the main menu.
For the first-time GUI programmer, this procedure might be a little overwhelming, but really it couldn't be simpler. The various System.Windows.Forms methods take care of adding GUI functionality to the menu and the items, so that when you compile and run the code, the File menu will function as it does in any GUI application: First you click to display the items, and then you click to fold them back in.
Of course, you still have to associate your menu with your original form code and program event handlers for the various items to get them to work. Also, you'll have to tie these event handlers to the particular menu item's click event. Further on, you have to create dialog boxes and add functionality to them with the use of buttons to save the file and do other tasks.
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
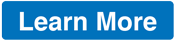
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.