Expandables
DIY Text Expander
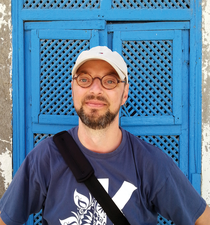
A couple of utilities and a dash of Bash scripting are all you need to roll out a simple yet flexible text expander.
A tool that can expand abbreviations and insert ready-made text snippets can come in handy in many situations. With a bit of Bash scripting and a couple of existing utilities, you can easily build your own text expander and learn a few clever techniques in the process.
The starting point of this project is a simple Bash script published on the Arch Linux forum [1]. This nifty script uses a combination of the XSel [2] and xdotool [3] tools to replace an abbreviation with the related text snippet (Listing 1). The xdotool, which simulates keyboard input and mouse activity, is used to select and cut the abbreviation text (by simulating the Ctrl+Shift+Left Arrow and Ctrl+X keyboard shortcuts).
The cut abbreviation is then set as the X selection with the XSel tool. The script then fetches the appropriate text file and copies its contents as the X selection. Finally, the xdotool pastes the copied contents by simulating the Ctrl+V keyboard shortcut. So, when you type foo
and run the script, it replaces the abbreviation with the contents of the ~/.snippy/foo
text file.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
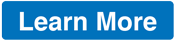
News
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.
-
Linux Kernel 6.15 Now Available
The latest Linux kernel is now available with several new features/improvements and the usual bug fixes.
-
Microsoft Makes Surprising WSL Announcement
In a move that might surprise some users, Microsoft has made Windows Subsystem for Linux open source.
-
Red Hat Releases RHEL 10 Early
Red Hat quietly rolled out the official release of RHEL 10.0 a bit early.
-
openSUSE Joins End of 10
openSUSE has decided to not only join the End of 10 movement but it also will no longer support the Deepin Desktop Environment.
-
New Version of Flatpak Released
Flatpak 1.16.1 is now available as the latest, stable version with various improvements.
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.