Writing web applications in Clojure
Functional Web
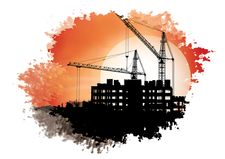
Clojure looks like Lisp and runs wherever Java is installed. Practical tools and sophisticated libraries provide the underpinnings for rapid development of modern web applications.
Clojure [1] made its first appearance in the software landscape in 2007. The functional programming language combines Lisp-like syntax with an implementation on the Java Virtual Machine (JVM). Within a few years, a Clojure community with user groups [2] and conferences (e.g., Clojure West [3] and Clojure/conj [4]) has been established.
An extensive ecosystem of libraries has emerged for the language, which is released under the Eclipse Public License. Clojure benefits from its Java origins, which lets it share the VM with Java and JRuby, and impresses with abstraction over concurrency [5] on multiprocessor systems. The name evokes the closure programming concept, with the "j" coming from Java.
Clojure advocates are enthusiastic about the improved productivity this expression-rich language permits. I strongly encourage you to take a look at an essay by Lisp advocate and entrepreneur Paul Graham [6] that describes the implications of the advantages Lisp programming confers, leading some Internet startups to focus on the language they use in large web applications. In this article, I show you how to use Clojure to implement a simple chat for the web browser (Figure 1).
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
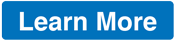
News
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.