How Perl programmers efficiently manage asynchronous program flows
Pyramid of Doom
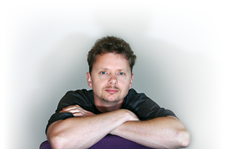
Asynchronous program flow quickly degenerates into unreadable code if it lacks an overarching concept to provide structure. Fortunately, the JavaScript community has invented some functional tricks that also help tame asynchronous Perl code.
Even experts can unintentionally build race conditions into their code. Even they can easily overlook the side effects when multithreading suddenly interrupts the flow of code. Emergency teams later have to discover the causes, which involves a huge amount of effort. After all, the error the customer reports occurs only sporadically, because it only occurs given the temporal coincidence of certain events that cannot be easily reproduced by the development department.
One Thread Is Enough
If only one thread is running – as in a JavaScript application – then there can be no such race conditions, because the CPU runs the code as written, and nothing unexpected interrupts the flow. In other words, this approach has its benefits.
For a program with only one thread to run as fast as one with multiple threads, however, it must not be allowed to sit and twiddle its thumbs while, for example, a much slower network operation is in progress. To allow this to happen, the programmer relinquishes control over selected parts of the program to an event loop, which executes the events asynchronously. Once a programmer's brain has mastered the jump to asynchronous program flows, even complicated processes are wonderfully easy to write. Before that happens, however, some hurdles must be taken.
Hipster, Help!
Some people take a long time to understand asynchronous program flow. The resurrection of JavaScript as a hipster language, especially on the server side with the fully asynchronous Node.js framework, brought to light some of the familiar and typical stumbling blocks. Some elegant solutions (e.g., PubSub, Promises, or entire frameworks like Async.js) have lately emerged to help tame the asynchronous flow of complex applications [1].
Asynchronous flow is something that newcomers also perceive as a limitation in Perl, because all of a sudden they cannot, for example, easily retrieve the content of a website with the LWP::User-Agent CPAN module and a call such as:
$ua->get("http://foo.com");
Because get()
is waiting for the response from the web server, and the data is slowly trickling in, all of the application cogwheels are standing still, which is truly undesirable if you want snappy performance. Instead, everything now relies on callbacks, as in the following example with the AnyEvent framework:
http_get("http://foo.com", sub { print $_[1] } );
The program simply issues the order to get the website and returns to the main program immediately after calling the http_get()
function. The event loop has accepted the job and only takes care of retrieving and collecting the data when the program again takes a break. Once everything is in place, the global event loop calls the previously attached callback with the acquired data, which it outputs using the print
statement.
However, this structure really messes up the program flow of an application:
http_get($url1, sub { http_get($url2, sub { # ... }); });
If multiple web requests occur in quick succession, whose queries involve previously retrieved results, nesting can quickly take on dimensions that are difficult to understand.
Pyramid of Doom
If not handled correctly, the callback method quickly leads to what is known as the "Pyramid of Doom." This refers to nested functions, where each callback defines another callback. At some point, even the widest screen is no longer wide enough to write out the program flow, not to mention the fact that such code is extremely difficult to read and comprehend. Who would argue with this statement given Listing 1?
Listing 1
http-get-nested
This simulates the problem that arises when the result of a web request flows into the next request, that is, where several web requests depend on one another. They can be processed asynchronously but need to keep to a predetermined order. To illustrate this, the script in Listing 1 [2] uses CountServer
, a test server, which responds to requests on the URL http://localhost:9090 by returning another URL – in each case with a numerical path component incremented by 1:
$ curl http://localhost:9090/test-1.txt http://localhost:9090/test-2.txt $ curl http://localhost:9090/test-2.txt http://localhost:9090/test-3.txt $ curl http://localhost:9090/test-5.txt http://localhost:9090/test-6.txt
The test client in Listing 1 starts the first asynchronous web request with the string /test-1.txt
; in response, it receives the string /test-2.txt
, which then sends the second request and receives test-3.txt
in return. The actual output from http-get-nested
in Listing 1 is:
$ ./http-get-nested Got: http://localhost:9090/test-2.txt Got: http://localhost:9090/test-3.txt Got: http://localhost:9090/test-4.txt
This confirms that although the code is quite difficult to understand, it is still quite functional. The CountServer.pm
code shown in Listing 2 is a very easy and simple Perl class whose start()
method launches a web server from the CPAN AnyEvent::HTTPD module. The reg_cb()
in line 22 lets the web server register a handler for incoming requests and thus return the path information to the requesting web client incremented by precisely 1 in each case.
Listing 2
CountServer.pm
If you're used to traditional sequenced programming and think you need to launch an external web server to test a web client, you may be scratching your head by now and suspecting some kind of sorcery. In the asynchronous world, however, it is common practice to run both server and client for testing purposes at the same time, in same program. This converts a unit test into an integration test!
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
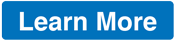
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.