CoffeeScript, Dart, Elm, and TypeScript
Script Mods
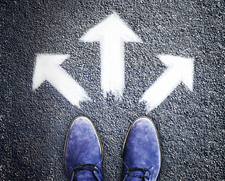
© Lead Image © Daniil Peshkov, 123RF.com
JavaScript is the stuff of which many interactive web clients is made, but it comes with a fair amount of historical ballast. The creators of four alternative scripting languages seek to ditch the ballast.
Browser-based interactive applications have helped the recent comeback of JavaScript, although the scripting language first saw the light of day in the mid-1990s. Over the course of time, JavaScript's makers added more and more new constructs to what was initially a fairly simple scripting language. One prime example is prototype-based object orientation, hated by many developers and often misunderstood and unused.
Not until 2015 was the scripting language, by then standardized as ECMAScript, given optional class-based object orientation. However, compared with Java, C++, and others, it still lacks certain features [1]; moreover, neither JavaScript nor ECMAScript offers type checking, which continually results in exception errors in practice.
For this reason, several scripting languages want to replace JavaScript – or at least simplify it in terms of programming. Some of the most widespread and popular examples are CoffeeScript, Google's Dart, Elm, and Microsoft's TypeScript. In a comparison, I describe what distinguishes the four candidates and in which areas they are superior to JavaScript.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
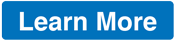
News
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.