Use a general purpose input/output interface on Linux computers and laptops.
Pinned
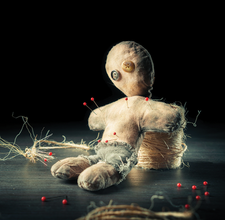
© Lead Image © Fernando Gregory Milan, 123RF.com
The general purpose input/output interface is not just for small-board computers anymore: You can use GPIO on your Linux desktop or laptop, too, through the USB port.
I am impressed and intrigued by the GPIO (general purpose input/output) capability of the Raspberry Pi. The GPIO makes it easy for a Raspberry Pi user to control real-world electronic gadgets like lights and servomotors.
Wouldn't it be great if you could do the same thing with an everyday Linux PC? You can. The Future Technology Devices International Ltd. (FTDI) FT232H device [1] provides GPIO capabilities (as well as various serial protocols) to regular, non-Raspberry Pi Linux desktops and laptops through a USB port. You can buy the FT232H device from Adafruit for $15.
In a previous issue of this magazine, I developed a simple application for the Pi that used the GPIO [2]. In this article, I rewrite my Raspberry Pi application to run on Intel desktop and laptop systems with the help of the FTDI FT232H device. This simple example will give you a taste for how you can use the FT232H to bring GPIO capabilities to a standard, Intel-based Linux computer.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
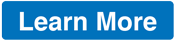
News
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.