Microcontroller programming with BBC micro:bit
Pocket-Size Programming
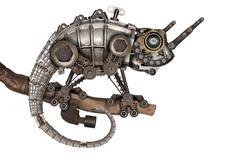
© Lead Image © Burmakin Andrey, 123rf.com
Designed for students, the BBC micro:bit, in conjunction with MicroPython and the Mu editor, can help you get started with microcontroller programming.
The idea of developing a microcontroller for schools dates back to 2012. In 2016, in cooperation with the University of Lancaster and several dozen industry partners, the British Broadcasting Corporation (BBC) delivered on this concept with the BBC micro:bit [1]. Although developed for seventh grade students, the BBC micro:bit offers an introduction to microcontroller programing for users of any age.
You can purchase the micro:bit individually for $14.95 or as the micro:bit Go Bundle (which includes batteries, a battery holder, and USB cable) for $17.50 from Adafruit [2].
The microcontroller and its components fit on a 5x4cm board (Figure 1). The 32-bit processor, an ARM Cortex-M0, runs at a clock speed of 16MHz. The micro:bit offers 16KB of RAM and a 256KB flash memory. In principle, a Bluetooth Low Energy (BLE) radio is also available. See Table 1 for more specifications.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
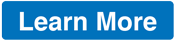
News
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.