Recursion
PHPWomen Contest Winner
ByPHPWomen.org recently held an article-writing contest on their Best Practices Forum. Authors of the two winning submissions each received copies of Zend Studio for Eclipse, a 1-year subscription to Linux Pro Magazine (which is called Linux Magazine outside North America), and the opportunity to feature their articles on the magazine websites. Congratulations goes to Rob Allen for his winning submission!
Recursion is the term used when an operation is repeated on the results of the same operation. That's a little confusing, but what it means to a programmer is that you have a function that calls itself.
Consider the process of adding up numbers in an array such as:
<?php
$array = array(10, 21, 4);
?>
All we need to do is iterate over the array and add each number to a variable as shown here:
<?php
function sumArray($array)
{
$total = 0;
foreach ($array as $element) {
$total += $element;
}
return $total;
}
$array = array(10, 21, 4);
$result = sumArray($array);
echo "result = $result\n";
?>
As you would expect, the output of the script is:
result = 35
But, what if the array is nested? For example, an array like this:
<?php
$array = array(10, 20, 5,
array(5, 2, 3)
);
?>
As we iterate over this new array, we will come to an element that is itself an array. We need to sum up the elements within this sub-array and, fortunately, we have just written a function that does just that (sumArray() !), so let's call it within the foreach() loop:
<?php
function sumArray($array)
{
$total = 0;
foreach ($array as $element) {
if(is_array($element)) {
$total += sumArray($element);
} else {
$total += $element;
}
}
return $total;
}
?>
This is recursion as we have called the sumArray() function from within the sumArray() function itself. That is, sumArray() is a recursive function.
We can now use our improved function to add up our nested array:
<?php
$array = array(10, 20, 5,
array(5, 2, 3)
);
$result = sumArray($array);
echo "result = $result\n";
?>
which will now output:
result = 45
As sumArray() is a recursive function, it will happily handle an array that is many nested levels deep. For example, consider an array that is nested 5 levels deep:
<?php
$array = array(10, 20, 5,
array(5, 2, 3,
array(5, 3,
array(2, 10,
array(19, 1)
),3
), 2, 7
), 3
);
$result = sumArray($array);
echo "result = $result\n";
?>
When this code is run, the output is:
result = 100
Clearly recursion is a powerful and flexible technique for solving problems involving nested data such as reading through XML structures or handling a tree within a database table (usually implemented with a parent_id column). It is also helpful when writing a sorting algorithm, but most PHP programmers don't need to do that! Recursive solutions also tend to be fairly compact and easy to debug as you only need to do it once!
Obviously, as we're programming, there are trade-offs involved! Recursive solutions are inefficient in terms of performance, so consider caching the result. Also, it's possible to get into situation where the recursion never stops. Always make sure that your function will end! Related to that, when you have deeply nested recursion, you can run out of "stack space" (this area reserved for the list of functions that are currently being called. In other words - make sure you test thoroughly :)
Go on.. write a recursive function today!
Comments
comments powered by DisqusSubscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
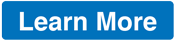
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
All pluses and no minuses ???