Speed up your web server with memcached distributed caching
Fast Cache
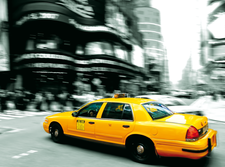
© gary718, 123RF
This practical caching tool can reduce the load on a web database server by as much as 90%.
Brad Fitzpatrick was frustrated: Although the LiveJournal.com blogger platform that he had founded – and for which he had done most of the development work – was up and running on more than 70 powerful machines, its performance still left much to be desired. Not even the database server cache size of up to 8GB seemed to help. Something had to be done, and quickly. The typical measures in scenarios like this are to generate some content up front or to cache pages that have been served up previously. Of course, these remedies require redundant storage of any elements that occur on multiple pages – a sure-fire way of bloating the cache with junk. If the systems ran out of RAM, things could be swapped out to disk, of course, but again this would be fairly slow.
In Fitzpatrick's opinion, the solution had to be a new and special kind of cache system – one that would store the individual objects on a page separately, thus avoiding slow disk access. Soon, he gave up searching for a suitable solution and designed his own cache. The servers that he wanted to use for this task had enough free RAM. At the same time, all the machines needed to access the cache simultaneously, and modified content had to be available to any user without any delay. These considerations finally led to memcached, which reduced the load on the LiveJournal database by an amazing 90 percent, while time accelerating page delivery speeds for users and improving the resource utilization on the individual machines.
Memcached [1] is a high-performance, distributed caching system. Although it is designed to be application-neutral for the most part, memcached is typically used to cache time-consuming database access in dynamic web applications.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
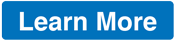
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.