Photo diary
DIY Photo Sharing
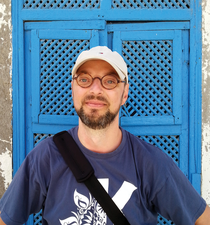
Instead of relying on a third-party service for instant photo sharing, you can build your own solution using existing software and a pinch of PHP and Python scripting.
Google+, Instagram, EyeEm – services for instant photo sharing – are plentiful. However, the broad choice of photo sharing services doesn't mean you can't build your own photo-sharing solution. In fact, if you are not fond of the idea that third-party services harvest and datamine your accounts, as well as use your photos for their own purposes, then going the DIY route makes a lot of sense.
Privacy concerns, however, are just one reason for deploying a home-brewed solution. By building your own instant photo-sharing application, you can focus on the features that are important to you, leaving out all the unnecessary embellishments. Creating your own app is also an excellent opportunity to master some basic scripting skills and write code that you can later reuse in other projects.
Building your own instant photo-sharing system doesn't have to be as daunting as it may sound, provided you are interested in creating a utilitarian solution rather than an Instagram killer. In this article, I'll guide you through the process of building a no-frills instant photo-sharing system that uses existing software and requires only a minimum of scripting. Creating and setting up the entire solution shouldn't take more than a couple of hours, which makes it a perfect project for a rainy weekend.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
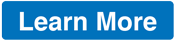
News
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.
-
Linux Kernel 6.15 Now Available
The latest Linux kernel is now available with several new features/improvements and the usual bug fixes.
-
Microsoft Makes Surprising WSL Announcement
In a move that might surprise some users, Microsoft has made Windows Subsystem for Linux open source.
-
Red Hat Releases RHEL 10 Early
Red Hat quietly rolled out the official release of RHEL 10.0 a bit early.
-
openSUSE Joins End of 10
openSUSE has decided to not only join the End of 10 movement but it also will no longer support the Deepin Desktop Environment.
-
New Version of Flatpak Released
Flatpak 1.16.1 is now available as the latest, stable version with various improvements.
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.