Web programming with ECMAScript 6
New Script
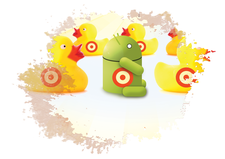
The new ECMAScript 6 language eliminates many historical problems associated with JavaScript.
In 1995, Netscape and Sun Microsystems announced the official arrival of the JavaScript scripting language. According to the original press release, JavaScript was intended as "… an open, cross-platform object scripting language for the creation and customization of applications on enterprise networks and the Internet." [1] The goal was to create a scripting language for the emerging HTML-based Internet that would run easily in the browser client context and would also have uses on the server side. The subsequent browser wars led to many battles over the role of JavaScript as a core technology for the Internet. Microsoft developed its own JavaScript-like language, which they called JScript, and the two implementations bore a striking resemblance but were just different enough to cause problems for web developers trying to write cross-platform programs.
The ECMA-262 specification emerged as an attempt to standardize JavaScript-like languages, so programmers would be able to operate independently of a single vendor. The programming language standardized in the ECMA-262 specification came to be known as ECMAScript [2]. ECMAScript still exists as a universal JavaScript-like language tailored for web development environments. The ECMAScript project website even calls ECMAScript "the language of the web," [3] and the standard is still an important means for understanding and predicting the evolution of web technologies.
JavaScript, JScript, and other alternatives such as Adobe's ActionScript, all offer compatibility with ECMA-262 and the universal ECMAScript language. The current version of the language is ECMAScript version 5, but version 6 is already available in draft form, and support for ECMAScript 6 is starting to appear in many popular browsers. Version 6 actually addresses some problems associated with contemporary versions of JavaScript
This article offers a quick preview for what you'll find in ECMAScript 6. I'll summarize some new features that will one day make their way into JavaScript and other compatible languages. I'll also describe a sample application – a metronome for the browser [4] created with the help of ECMAScript 6, the Traceur compiler, HTML5, the Web Audio API, and CSS3.
New Language Resources
ECMAScript 6 hardly invents anything new; instead, it resorts to tried and tested assets from other languages. For example, the new version with arrow functions introduces Lambda functions (known from, e.g., Haskell). They reduce the amount of typing for function definitions and lexically bind the special this
variable. In the expression
[2,4,6,8].map(x => x+1)
x => x+1
describes a callback function that, up to now, programmers could only define as function(x) {return x+1}
.
Familiar constants from C are also new. The const
keyword introduces the definition of constants in ECMAScript 6; for example, const c = 7;
. The value of constants, as in C, cannot be overwritten.
The let
keyword at the beginning of a variable definition, for example let x = 2;
, restricts the visibility of the variables to the surrounding block. Listing 1 contains let
in the header of a for
loop in the first line. The x
variable defined by let
is undefined outside the loop. If the keyword var
were used in place of let
, x
in line 4 would have a value of 1
.
Listing 1
let-statement.html
Class Conscious
ECMAScript 6 for the first time offers the ability to create objects using a class in a fashion similar to Java. Listing 2 shows the definition of two classes as per the new standard. The class
keyword introduces the definition for the Creature
class in line 1, which is followed by the class constructor function (lines 2-5) with the body surrounded by curly brackets. The function is run when creating an object, as in
Listing 2
class-statement.html
new Creature('Bob', 'friendly')
The constructor function assigns the values of the name
and age
call parameters from line 2 to the name
and age
attributes in lines 3 and 4.
Inheritance
The definition of the class Human
(lines 8-17) is derived from the Creature
class using the extends
keyword. The constructor function from Human
calls the parent class constructor function in line 10 using the super()
function. Instances of Human
thus inherit the properties name
and age
.
Human
also contains the toString()
method. Its definition begins with the name followed by an empty parameter list and ends with the function block in curly brackets. The toString()
block creates a representation of the object as a string. The method also uses a string literal expression in line 15.
String literals are also new in ECMAScript 6. Quoted in backticks, the parameter expansion occurs in the form ${<Variable name}>}
– in a similar style to Bash. To test it, line 19 evaluates the expression
(new Human('Bob', 3, 'friendly')).toString()
The log()
method writes the result Bob (3) is a friendly human to the browser console.
As with Node.js, developers can outsource functionality into modules with ECMAScript 6. Listing 3 first shows a module. The export
keyword causes the module to export the pub
variable in line 2 and the pubObj
class in line 3. The priv
variable from line 1, on the other hand, remains internal. Only the module itself can access it, as in line 5.
Listing 3
export-statement.js
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
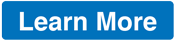
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.