Zack's Kernel News
Zack's Kernel News
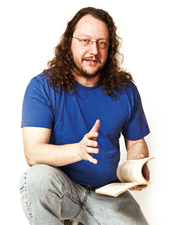
Chronicler Zack Brown reports on the latest news, views, dilemmas, and developments within the Linux kernel community.
Bogus GCC Warnings Reveal Real Kernel Problem
Linus Torvalds recently took great pains to explain why even though a particular GCC warning resulted in identifying a true problem with the kernel code, the warning itself was nevertheless bogus and insane. In fact, he said, most of the warnings introduced in GCC 5.1 didn't seem so valuable to him.
The one that had caught his attention, though, was a warning against using a switch
statement with a Boolean variable. Presumably, the GCC folks thought that the whole point of switch
was to operate on several possible values using a simple coding structure, and thus avoid long if
/else
/if
chains. They may have thought that for a Boolean variable, a simple if
statement would be the only appropriate way to go.
In the case of this particular bit of kernel code, said Linus, the warning was good because the switch
statement in the kernel used a Boolean variable, whereas the case
statements did not. He felt that this would be a perfectly legitimate thing for GCC to issue warnings about. But, if the switch
and case
statements both used a Boolean variable, he said, it would be a perfectly fine use case, and might even be better than the equivalent if
/else
in some cases:
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
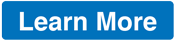
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.