Node-RED basics for controlling IoT devices
Go with the Flow
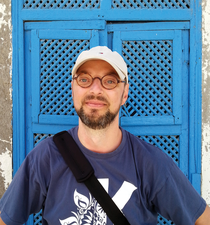
Learn how to use Node-RED to automate tasks, work with web services, and do other clever things.
Just as an orchestra needs a conductor, Internet of Things (IoT) devices and web services need a tool that wires them together, defines their roles, and specifies rules for their behavior. This is essentially what Node-RED [1] does. Built by IBM, this open source Node.js-based application provides a graphical environment for building flows – simple and complex programs that tie various devices and services together, as well as manipulate and move data between them.
This functionality makes it possible to automate various tasks and program devices and services by connecting Node-RED modules called nodes and adding a dash of JavaScript code. For example, you can easily create a simple flow that pulls and processes weather data from the OpenWeatherMap service and sends daily weather reports to a specified email address. It is also possible to set up a flow that reads data from sensors connected to a Raspberry Pi or Particle's Photon WiFi board and pushes the obtained data to a Google Docs spreadsheet or Twitter. To automate tasks and orchestrate IoT devices and services, you need to master Node-RED's basics, and in this article, I will help you to get started with this powerful and versatile application.
Installing Node-RED
Although Node-RED can run on a regular Linux computer, you might want to use a dedicated machine to act as a Node-RED server (see the "Using FRED" box for more options); a low-cost single-board computer like Raspberry Pi is perfect for that. In fact, the latest version of Raspbian based on Debian Jessie has Node-RED preinstalled, so you don't even need to spend time deploying the application.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
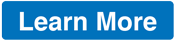
News
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.