From disk to paper
Tutorial – Printing in the Shell
A few commands and some simple shell scripts make it easier to manage your printer so that you can access print functions quickly and automate recurring tasks.
If you work with LibreOffice or an image processing program like Gimp, you don't have to look too hard for the print function. The print icon is usually located in the upper left corner of the buttonbar; alternatively, you can press Ctrl+P. In many situations, however, it would be more practical to print without the help of an application – for example, if you want to print from a script.
Complex printing commands can also be transferred as shell commands. There are instructions to fit several pages on one sheet, for duplex printing, for cover sheets to make sorting easier, or for options to change the page orientation.
Linux basically comes with two commands for controlling printers at the command line, lp
and lpr
. Table 1 shows some important options, while Table 2 lists some helpful variants for everyday use. For additional options and settings, check out the extensive man pages for lp
[1] and lpr
[2].
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
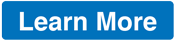
News
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.