GCC, Clang, and MSVC compilers with C++
Perfect Match
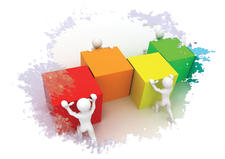
Due to the fast pace of updates of the C++ standards, compiler builders have been busy. How do the most popular compilers fit with the standards and what are the differences?
The quiet times are over for C++. A full 13 years passed between the C++98 and C++11 standards, but since then, new standards have appeared every three years, with C++14, C++17, and preliminary work on C++20. The C++ standardization committee already shows signs of enthusiasm for the next cycle.
With so many versions of C++ out in the world (see the "Blessings of Diversity" box), a developer could easily wonder, how do the compiler makers keep up with it all? This article looks at support for C++ standards in three popular compiler alternatives:
- GCC: The GNU Compiler Collection (GCC) [1] is the quintessential free software compiler. Originally created by Richard Stallman in 1987 as the compiler for the GNU project, GCC is now supported by a large community of developers and is found on almost all Linux distributions.
- Clang: This popular free compiler collection [2] uses the LLVM compiler as a back end. Clang is actually the front-end component. Clang/LLVM is designed for a high degree of compatibility with GCC. The Clang project enjoys the support of several major vendors, including Apple, Google, Microsoft, Intel, and AMD, possibly because they find Clang's permissive free software license easier to integrate with commercial projects than the GPL3 license attached to GCC.
- MSVC: This compiler is for Microsoft's Visual C++ (MSVC) environment [3]. In the past, Linux support in a major Microsoft development tool would have been unthinkable, but today, versions of MSVC run on Linux, and extensions are available for developing Linux applications on MSVC.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
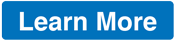
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.