Organizing and reusing Bash code
Tutorials – Bash Functions
Learn how to make your Bash code more readable, robust, and reusable by managing the code within your Bash scripts.
A common problem with every scripting or programming language is that the more complex you make your code, the more difficult it is to understand, debug, and extend that code – unless you organize it correctly from the beginning.
In this installment of my shell scripting series, I show how Bash can help you organize your code correctly along with some related best practices. With a focus on how and why to use Bash functions and variable scopes, I'll present a shell script that can load code from other files, plus a few practical examples.
The Basics
Bash functions allow you to wrap up, as a single command, more or less complex chunks of code that perform any kind of task. You can then invoke these functions as many times as you like inside your script. Bash provides two equivalent syntaxes for defining functions. The first syntax consists of using the function
keyword, followed by the function name, and finally curly braces that enclose all the function's code:
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
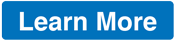
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.