Writing IRC bots with Perl's BasicBot
Chat Bot
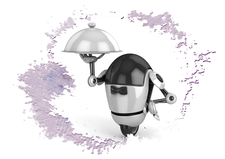
Writing an IRC chat bot does not have to be difficult. We'll show you how to create your own custom IRC bot using the Perl BasicBot module.
Internet Relay Chat (IRC) is a popular protocol for sustaining group conversations online. The user connects to an IRC server, which is usually part of an IRC network, and joins chat rooms, known as channels, in IRC terminology. Channels are carried by every server that is part of the same IRC network, so users all across the network can join the same channels and talk to each other in real time.
An IRC bot is a program that connects to a chat service as if it were a user and performs automated tasks. Bots are used for running games, conveying messages across different networks, managing user authentication, and much more.
Building a custom bot might seem like a high-end task limited to an enterprise web portal or other commercial service, but in fact, homegrown bots serve a number of roles on smaller networks and are often deployed by local organizations and community groups. For instance, the #bitcoin-otc
channel in the Freenode IRC network makes use of a bot for maintaining the reputation score of the buyers and sellers that trade in the chat. On the other hand, the I2P project keeps a bot that passes messages from users in Freenode to users in Irc2p, the official I2P IRC network.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
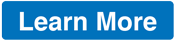
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.