Distributed weather monitoring in gardens
As any gardener knows, an understanding of local weather plays an important role in gardening success. Frost can kill off delicate seedlings, too much sun can frazzle shade-loving plants, and too much moisture can suffocate roots. I have relied for a few years on a single temperature sensor outside and an indicator inside for an overview of the conditions outdoors. Although it is a useful tool, it has several drawbacks: The wired sensor is at a single, fixed location, only measures current temperature, and is too close to the house. I wanted a system that provides multiple measurement types at multiple locations, requires no wires trailing around the garden, would provide seasonal data to better plan for planting, and has the capacity for alarms to warn of frost and high temperatures. Ideally, the data would be available from a computer and mobile phone without installing additional software.
In this article, I describe the design and implementation of such a system with only free and open source tools in a Linux environment. With this system, I hope to identify microclimates within my garden and select optimum planting conditions for plants with different needs.
Multiparameter Sensor
The most important requirement for a sensor for this application is that it transmits data wirelessly, which, of course, implies battery power. I considered a number of wireless technologies, including WiFi and Bluetooth, but I rejected these on the basis of power consumption, limited range, and other reasons. I've worked previously with LoRa [1], a radio technology expressly designed for low data rate, low power, and long-range data telemetry. Additionally, the LoRaWAN wide area network protocol works with a network of public gateways and public "landing points" for data produced by sensors. This protocol exists expressly to build networks of Internet of Things (IoT) sensors.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
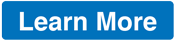
News
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.