Write Inkscape extensions that modify objects
Tutorial – Tremble
Writing your own extension for Inkscape opens up a whole world of possibilities. Apart from creating new objects, you can modify existing objects and even animate them.
In last month's issue [1], we saw how to write an extension that rendered an object (a circle) in an Inkscape document. But apart from creating new objects, Inkscape extensions can also be used to modify existing objects. Let's see how this can be done be creating an extension that will generate "wobbly" animation. The idea is to take a given path – say, a piece of text – and to move its nodes around a little bit. Then save the result as a frame, move the nodes a little more, save again, and so on. When you put the frames together, it will give the impression that the text wobbles. This video shows how to do it in After Effects [2], but it seems like a lot of work for something that could be done with a relatively simple script.
Unfortunately, documentation and tutorials explaining how to create this type of script are all but nonexistent. Again the only way forward is to wade through source code and comments within code [3] to try and figure out what tools are available to achieve our ends.
What Is a Node?
Any object in Inkscape can be broken down into a bunch of paths, and paths, in turn, are made up by a bunch of nodes. So the key to modifying any object is modifying its nodes. But what is a node? You may think it is the point on a path where the path can change direction. In fact, that is just one control point, and a node is made up of three control points.
[...]
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
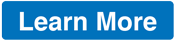
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.