Anatomy of a kernel attack
Overflow
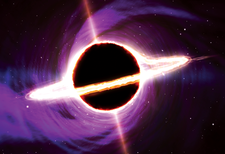
A vulnerability in an operating system kernel is a security nightmare. This article analyzes some well known kernel security problems, explains how they are exploited, and gives real-life examples of attacks that used these time-honored techniques.
Some security issues remind me of Groundhog Day – they just keep coming back. One example of a problem that won't go away is buffer overflow, which was first described in 1972 [1], got a fair bit of attention in 1996 in the oft-quoted Phrack e-zine article "Smashing the Stack for Fun and Profit" [2], and is still one of the most prevalent programming mistakes that can lead to a code injection. And it isn't due to the lack of media coverage that integer overflows are still around – long after they caused the Ariane 5 rocket explosion [3] and the massive Stagefright security issue in Android. The fact is that many of the same few problems continue to turn up, and most could have been prevented by code analysis [4] and defensive programming.
This article takes a close look at some of the techniques attackers use to crack the Linux kernel.
Stein and Shot
You can perform a simple test at home for a graphic example of a buffer overflow: Grab a shot glass and a comfortably sized Munich beer stein, fill the stein to the top, then pour all of its contents into the shot glass. Take note of the overflow. To prevent this situation, the bartender must compare the source's size to the size of the destination buffer.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
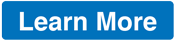
News
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.