Harder than scripting, but easier than programming in C
Programming Snapshot – Golang
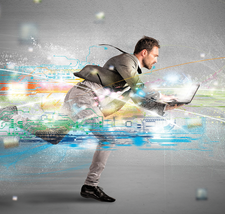
© Lead Image © alphaspirit, 123RF.com
Released back in 2012, Go flew under the radar for a long time until showcase projects such as Docker pushed its popularity. Today, Go has become the language of choice of many system programmers.
In 2012, Unix and C veterans Robert Griesemer, Rob Pike, and Ken Thompson released the system-oriented programming language Go under the aegis of Google. For a long time it eked out a niche existence, before eventually becoming the industry standard for system-oriented programming. Today, observers of the Unix scene are rubbing their eyes in disbelief over the number of tools developed in Go.
To name a programming language after an everyday word such as Go seems like a pretty crazy idea from the viewpoint of a search engine operator. After all, search engines actually remove filler words such as "go" from incoming queries. So, when looking for Go programming tips, the recommendation is to search for "Golang" instead, which has also become the accepted name for the language in the community.
Quickly Installed
If you want to try Go, the easiest approach is to grab a package for your favorite distro. On Ubuntu, for example, type:
sudo apt install golang
After the install, go build
gives you a super-fast Go compiler; gofmt
a pretty printer; go doc
a renderer for manual pages, an extensive core library, and much more.
Go offers a mature development framework, a huge standard library for handling typical programming tasks, support for automatic testing, and a lively community that keeps uploading new libraries to GitHub, from which you can easily include them in your own applications via simple references in your own code.
In the following exploration, I'm going to present only a limited number of useful language features; many may sound familiar, as you might have heard of them in typical "language wars" in the vein of endless discussions such as "Emacs vs. vi". If you want to learn more, I recommend Go's online interactive tutorial [1] or the excellent original book written by one of the Go makers [2].
gofmt
The Internet community has wasted a good deal of energy over the years infinitely discussing the right number of indentations or spaces between keywords or even the "right" editor.
Following Python's strict example to some extent, the Go community prefers a very specific code format. The compiler doesn't grumble if someone now uses four or eight spaces for indentation instead of tabs, but the community insists on principle that any code is run through the gofmt
prettyfier first before it appears anywhere online in a repository such as GitHub. The formatter rigorously sets tabs for indentation and removes spaces between round brackets and text, but puts spaces around punctuation such as +
or =
for easier reading. There is no arguing with this; it is just the way things are done.
The situation is similar for CamelCase within variables and functions: geoSearch()
is in; geo_search()
is out. By the way, it also matters whether you start a variable with upper or lowercase. In a structure or package, Go exports uppercase variables outside the current context, and lowercase variables remain private.
Result or Error?
One hot topic in programming languages is the pros and cons of exceptions (Java, Python) versus returned error codes. Go sees itself in the tradition of the classics like C (which is understandable given Go's list of authors) and evaluates return values with each function call – but with a twist. Since Go functions can return several values, an error code usually comes back along with a result, like with the ReadFile()
function from the Go os
standard library, for example (see Listing 1 [3]).
Listing 1
readfile.go
package main import ( "log" "os" ) func main() { data, err := os.ReadFile("/tmp/dat") if err != nil { log.Fatal(err) } os.Stdout.Write(data) }
The first return value, data
, contains the data found inside the specified file, as an array of the type []byte
after a successful read operation. However, if something goes wrong, an error is returned as the second value in the err
variable. The main program code checks this result as instructed with err != nil
. This returns a true value for the if
condition in case of an error because, in case of success, err
is set to nil
.
By the way, in case of an error, you should not use the other return value (data
). Usually, it is set to its so-called null value in Go anyway, which variables assume after they are declared but not yet initialized. In the example of an array of type []byte
, this is an empty array.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
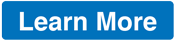
News
-
NVIDIA Released Driver for Upcoming NVIDIA 560 GPU for Linux
Not only has NVIDIA released the driver for its upcoming CPU series, it's the first release that defaults to using open-source GPU kernel modules.
-
OpenMandriva Lx 24.07 Released
If you’re into rolling release Linux distributions, OpenMandriva ROME has a new snapshot with a new kernel.
-
Kernel 6.10 Available for General Usage
Linus Torvalds has released the 6.10 kernel and it includes significant performance increases for Intel Core hybrid systems and more.
-
TUXEDO Computers Releases InfinityBook Pro 14 Gen9 Laptop
Sporting either AMD or Intel CPUs, the TUXEDO InfinityBook Pro 14 is an extremely compact, lightweight, sturdy powerhouse.
-
Google Extends Support for Linux Kernels Used for Android
Because the LTS Linux kernel releases are so important to Android, Google has decided to extend the support period beyond that offered by the kernel development team.
-
Linux Mint 22 Stable Delayed
If you're anxious about getting your hands on the stable release of Linux Mint 22, it looks as if you're going to have to wait a bit longer.
-
Nitrux 3.5.1 Available for Install
The latest version of the immutable, systemd-free distribution includes an updated kernel and NVIDIA driver.
-
Debian 12.6 Released with Plenty of Bug Fixes and Updates
The sixth update to Debian "Bookworm" is all about security mitigations and making adjustments for some "serious problems."
-
Canonical Offers 12-Year LTS for Open Source Docker Images
Canonical is expanding its LTS offering to reach beyond the DEB packages with a new distro-less Docker image.
-
Plasma Desktop 6.1 Released with Several Enhancements
If you're a fan of Plasma Desktop, you should be excited about this new point release.