Create a digital spirit level with the ESP32
To build a digital spirit level, you first need a sensor such as the MPU6050, which determines the position of an object in space. It has an accelerometer and a gyroscope for each axis in space and measures just 4x4 millimeters. For our test setup, we used a module to hold the semiconductor. You can get it from AZ-Delivery for EUR4.79 [1]. If it is sold-out there, you can also purchase the module on Amazon or from Reichelt.
The MPU6050 communicates with the Raspberry Pi via the I2C bus. The AD0 connector defines whether the sensor resides on bus address 0x68
(AD0 to GND) or 0x69
(AD0 to VSS). The operating voltage for the module is in the range of 3.3 to 5 volts. If you need more information about the MPU6050, take a look at the datasheet [2]. To access the sensor, we will use a library with simple functions for accessing the sensor's readings.
I went for an ESP32, a highly integrated microcontroller with an unbeatable price/performance ratio, to process the data from the sensor. I will be using an ESP32 development kit [3], which you can pick up fairly cheaply for EUR9.49. The Arduino IDE provides the development environment.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
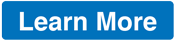
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.