Predicting the productivity of a solar array with Perl
Putting Everything Together
What you eventually want is for the computer to download a forecast reaching many days in the future and, taking your power consumption profile into account, give you an idea of how much surplus power you are going to get in future days. A good strategy is to have a cronjob fetch the forecast file from Solcast right after midnight and then parse it with a program capable of making these estimations. The script in Listing 3 is a proof of concept for such a program.
Listing 3
solar_2.pl
01 #!/usr/bin/perl 02 03 # Usage: ./solar_2.pl forecast.csv profile.csv $power 04 05 use DateTime; 06 07 my @csv_forecast; 08 my @csv_profile; 09 10 my $line_number = "0"; 11 open (my $fforecast, '<', $ARGV[0]) or die "Failed to open $ARGV[0]"; 12 while ( my $line = <$fforecast>) { 13 14 $line =~ s/\R/\012/; 15 chomp ($line); 16 17 my ($power,$timestamp) = (split "," , $line)[0,3]; 18 ${csv_forecast[$line_number]}{"power"} = $power; 19 ${csv_forecast[$line_number]}{"timestamp"} = $timestamp; 20 21 ++$line_number; 22 } 23 close ($fforecast); 24 25 $line_number = 0; 26 open (my $fprofile, '<', $ARGV[1]) or die "Failed to open $ARGV[1]"; 27 while ( my $line = <$fprofile>) { 28 29 chomp ($line); 30 31 my ($timestamp,$percentage) = (split "," , $line); 32 ${csv_profile[$line_number]}{"timestamp"} = $timestamp; 33 ${csv_profile[$line_number]}{"percentage"} = $percentage; 34 35 ++$line_number; 36 } 37 close ($fprofile); 38 39 # For each 30 minutes period, calculate whether we are going to produce 40 # more power than we are going to consume (and therefore waste it), 41 # or whether we are going to consume more power than we produce (and 42 # therefore have deficit). 43 44 my $waste = "0"; 45 my $deficit = "0"; 46 my $produced = "0"; 47 48 my ( $y,$m,$d,$h,$mt,$sec ); 49 my ( $i,$j ); 50 for ( $i = 1 ; $i < @csv_forecast ; ++$i ) { 51 52 ($y,$m,$d) = $csv_forecast[$i]{"timestamp"} =~ /^([\d]{4})\-([\d]{2})\-([\d]{2})/; 53 ($h,$mt,$sec) = $csv_forecast[$i]{"timestamp"} =~ /T([\d]{2})\:([\d]{2})\:([\d]{2})Z$/; 54 unless ( defined ($h) ) { ($h,$mt,$sec) = ( '0', '0', '0') }; 55 my $forecast_time = DateTime->new( 56 year => $y, 57 month => $m, 58 day => $d, 59 hour => $h, 60 minute => $mt, 61 second => $sec, 62 time_zone => 'UTC' 63 ); 64 $forecast_time->set_time_zone('local'); 65 66 my $consumption; 67 my ( $hour,$minute ); 68 for ( $j = 1 ; $j < @csv_profile ; ++$j ) { 69 ($hour,$minute) = $csv_profile[$j]{"timestamp"} =~ /([\d]{2})\:([\d]{2})$/; 70 71 if ( $hour == $forecast_time->hour() and $minute == $forecast_time->minute() ) { 72 $consumption = $csv_profile[$j]{"percentage"} * $ARGV[2] * 0.01; 73 } 74 75 } 76 if ( $consumption > ( $csv_forecast[$i]{"power"} * 0.5 )) { 77 $deficit += $consumption - ( $csv_forecast[$i]{"power"} * 0.5 ); 78 } else { 79 $waste += ( $csv_forecast[$i]{"power"} * 0.5 ) - $consumption; 80 } 81 82 $produced += $csv_forecast[$i]{"power"} * 0.5; 83 84 # If the day has changed, print information for the day. 85 if ( $forecast_time->hour() == "0" and $forecast_time->minute() == "0" ) { 86 $forecast_time->subtract( days=> "1"); 87 print "INFORMATION FOR DATE ". $forecast_time->date() . "\n"; 88 print "Total power produced will be $produced kWh\n"; 89 print "About $deficit kWh will be taken from the grid (deficit)\n"; 90 print "About $waste kWh will be produced but not used (wasted)\n\n"; 91 92 $deficit = 0; 93 $waste = 0; 94 $produced = 0; 95 } 96 } 97 98 exit;
The script assumes solar arrays are working without battery backup and attempts to guess how much power your house will take from the grid and how much power will be produced but not used (Figure 7). Power considered "wasted" is what would be sold to the power supply company if you sign a smart grid contract. This script iterates over a given forecast file and calculates the time period covered by the file.
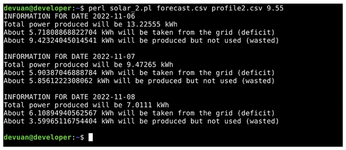
Lines 10-37 load the forecast and profile files to memory with the same approach as solar_1.pl
. The profile file needs no removal of Windows-style newline terminations; therefore, it lacks a replacement regex such as the one featured on line 14. Lines 50 to 96 iterate through all the lines of the CSV forecast file, discarding the first one (which does not store a useful value). Although not really recommended by Perl gurus, I decided to use a C-type loop for readability. Lines 52-54 use regular expressions to extract the year, month, day, hour, minute, and second corresponding to the timestamp of the entry of the forecast file. Lines 55-64 create a DateTime
object corresponding to the timestamp and adjust it to the local time zone.
Lines 68-75 look in the profile file for the entry corresponding to the timestamp it is currently analyzing and calculate the total power you expect the house to consume in that period. This calculation multiplies the total energy use expected for the day by the percentage of daily power use expected in the period corresponding to the timestamp, divided by 100. The result, in kilowatt-hours, is stored in the $consumption
variable.
The calculations in lines 76-80 are the energy debt and excess. If the house consumes more power than is produced, the script notes the energy debt in the $deficit
variable. Otherwise, excess power is noted in the $waste
variable. The script also records the power produced so far by the solar array during the day in line 82.
Line 85 guesses whether the end of the day has arrived. If so, the script prints the information corresponding to the day just parsed and sets $deficit
, $waste
, and $produced
to zero to start the next day anew.
Conclusion
The script in Listing 3 is a proof of concept and lacks input validation. It does not check whether the CSV files fed to it are correct and lacks appropriate exit statuses for input errors. However, it serves as a base on which to build a prediction system. You can easily set up a cronjob to download a forecast file from Solcast each day at midnight, generate a prediction with the script, and send it by email or post it to a website. The possibilities are endless (Figure 8).
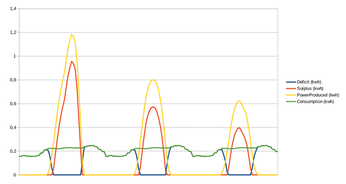
A more complex script that supports input validation and tracks the state of the battery banks is available at my gophersite [6].
Infos
- Solcast irradiance prediction service: https://solcast.com.au/
- IDAE residential consumption in Spain: https://www.idae.es/uploads/documentos/documentos_Documentacion_Basica_Residencial_Unido_c93da537.pdf (in Spanish)
- US EIA energy use in homes: https://www.eia.gov/energyexplained/use-of-energy/electricity-use-in-homes.php
- UK household energy usage: https://www.ukpower.co.uk/home_energy/average-household-gas-and-electricity-usage
- Power output of the Spanish power grid, February 2022: https://demanda.ree.es/visiona/peninsula/demandaqh/tablas/2022-11-2/1 (in Spanish)
- RubÈn Llorente's gopherhole: http://gopher://gopher.operationalsecurity.es/1/Software
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
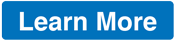
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.