Mozilla's systems programming language Rust
Traits
Traits are designed for code abstraction across data type borders. They ensure that developers use generic functions similarly to typeclasses of the Haskell [5] functional programming language.
Listing 3 creates the trait Dimension
, which in line 2 only sets the signature of the method volume()
. Implementations follow in line 9 for a sphere (data type Sphere
) and in line 15 for a cube (data type Cube
).
Listing 3
Body Calculations (trait.rs)
The signature (line 2) is relevant because it affects the method volume()
, which the code in lines 10-12 defines for the data type Sphere
. Line 11 calculates the sphere volume and reads the required radius from the field of the same name. The same approach is taken in lines 19-23 for the Cube
data type.
The program's main routine in lines 33-36 invokes the generic function print_volume()
with a value for the data types Sphere
and Cube
in each case. All values whose corresponding data type is implemented by Dimension
can be adopted here. The trait is named in line 25 after the abstract type variable T
and the colon.
If the program then invokes print_volume()
, the volume()
method runs against a matching data type. The println
macro outputs the results.
Macros
Macros generate code at compilation time that often greatly reduces programming effort. The programmer can use macros like println
very much like a function. However, an exclamation mark follows the name of a macro, as shown in line 8 of Listing 4.
Listing 4
Macros (macro.js)
Macros differ even more clearly from functions in terms of their syntax. The first five lines of Listing 4 show the code of the invoked macro length
. The name of the macro follows the keyword macro_rules!
.
Rust matches ($expression:expr)
for each call with the parameters transferred. The pattern is, however, so generic that it works for any value. At the same time, it stores the transferred value in the metavariable $expression
. The specification :expr
qualifies the value in the variable as a Rust expression.
If Rust notes a match while comparing, the block from line 2 evaluates it. In line 3, the stringify
macro converts the value from the metavariable to a character string whose length, as before, is output by println
. Anyone who compiles and executes the file macro.js
with
rustc macro.js && ./macro
will see the message The length of the expression is: 5 as the result.
Cargo
Like Gems [6] in Ruby or Pip [7] in Python, Rust also has its own package manager, Cargo [8], which generates and manages Rust packages at the command line. The Rust community currently offers around 2,200 finished packages [9] (Figure 1). The command
curl -sSf https://static.rust-lang.org/rustup.sh | sh
installs not only the latest stable binary version of the Rust compiler on Linux, but Cargo as well. The
cargo new test
command builds the directory structure shown in Listing 5 as a frame for the Rust package test
in the same folder.
Listing 5
Typical Directory Structure
The file Cargo.toml
(Listing 6) backs up the metadata for the package. GitHub describes the format of the Toml [10] language used in this specification. The configuration file contains the name, version, and the authors, where appropriate, as well as the package dependencies in INI style.
Listing 6
Cargo.toml
The file lib.rs
(Listing 7) contains the Rust code for the package, which you can include in your Rust programs later with the help of the assignment use test;
. You can then cd test
to enter the package directory and compile the project using cargo build
with the rustc
Rust compiler.
Listing 7
lib.rs
Using cargo test
(Figure 2), you can filter lib.rs
test functions such as gt_zero()
out of the package and then execute them. The Rust option #[test]
reveals gt_zero()
as the test function. Supported by the macro assert
, it generates the test result for the test 1>0
.
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
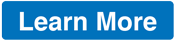
News
-
NVIDIA Released Driver for Upcoming NVIDIA 560 GPU for Linux
Not only has NVIDIA released the driver for its upcoming CPU series, it's the first release that defaults to using open-source GPU kernel modules.
-
OpenMandriva Lx 24.07 Released
If you’re into rolling release Linux distributions, OpenMandriva ROME has a new snapshot with a new kernel.
-
Kernel 6.10 Available for General Usage
Linus Torvalds has released the 6.10 kernel and it includes significant performance increases for Intel Core hybrid systems and more.
-
TUXEDO Computers Releases InfinityBook Pro 14 Gen9 Laptop
Sporting either AMD or Intel CPUs, the TUXEDO InfinityBook Pro 14 is an extremely compact, lightweight, sturdy powerhouse.
-
Google Extends Support for Linux Kernels Used for Android
Because the LTS Linux kernel releases are so important to Android, Google has decided to extend the support period beyond that offered by the kernel development team.
-
Linux Mint 22 Stable Delayed
If you're anxious about getting your hands on the stable release of Linux Mint 22, it looks as if you're going to have to wait a bit longer.
-
Nitrux 3.5.1 Available for Install
The latest version of the immutable, systemd-free distribution includes an updated kernel and NVIDIA driver.
-
Debian 12.6 Released with Plenty of Bug Fixes and Updates
The sixth update to Debian "Bookworm" is all about security mitigations and making adjustments for some "serious problems."
-
Canonical Offers 12-Year LTS for Open Source Docker Images
Canonical is expanding its LTS offering to reach beyond the DEB packages with a new distro-less Docker image.
-
Plasma Desktop 6.1 Released with Several Enhancements
If you're a fan of Plasma Desktop, you should be excited about this new point release.