Explore Fyne, a GUI framework for Go
My new favorite language, Go, impresses with its image processing routines, manipulating the pixels in an image just as quickly as ordinary numbers or text [1]. I often paint arrows in digital images, either for illustration purposes or to point out something funny in a snapshot, and I wondered how hard it would be to automate this task. Thus far, I have always had to fire up Gimp, select a path with the Path tool, and then place an arrow in the image with an Arrow plugin that I downloaded from somewhere on the Internet. With Go, there's got to be an easier way!
GUI Preferred
Actually, I prefer command-line tools, but sometimes a traditional graphical interface is just more practical, for example, to select a place in a photo where I want the program to draw a red arrow.
The arrow in Figure 1 illustrates, for example, that the tape measure in the photo, which I found while rummaging through my dusty desk drawers, is an age-old tchotchke from the once thriving company Netscape. Remember them? Yes, the browser manufacturer, where I worked for a few years during the 1990s, shortly after I left Munich and escaped to Silicon Valley "for a year" but somehow never found my way back.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
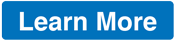
News
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.