Designing cross-platform GUI apps with Fyne
Platform Agnostic
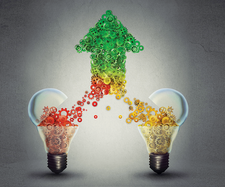
© Lead Image © Ion Chiosea, 123RF.com
The Fyne toolkit offers a simple way to build native apps that work across multiple platforms. We show you how to build a to-do list app to demonstrate Fyne's power.
One of the biggest challenges in modern app development is the requirement to develop a separate codebase for each platform that you want to support. Fortunately, the Fyne graphical user interface (GUI) toolkit lets you program your app once and then deploy it rapidly to multiple devices.
Fyne is an open source GUI toolkit built for the Go programming language, a popular general-purpose compiled programming language created by Google a little over 10 years ago. Go is frequently used for cloud services and infrastructure projects. However, the Fyne toolkit makes it possible to quickly build native GUIs for desktop and mobile devices. One of a number of GUI toolkits for Go, Fyne aims to be the simplest to use, appealing to both beginners and experienced GUI developers.
Fyne's idiomatic design makes it easy for Go developers to rapidly learn its principles. Fyne allows developers to create their own custom widgets thanks to its rich widget set and extensibility. With graphical elements inspired by Material Design, Fyne will feel familiar to many end users, while remaining open to theming by app developers. Fyne is fully unit tested and supports rigorous test-driven development. Released under the same permissive BSD licence as Go, Fyne is supported by an active development community.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
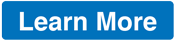
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.