Build a complete game with the Godot game engine
Writing a game from scratch is hard, and that's why nobody does it anymore. Game creators instead use "engines" that combine a framework and a comprehensive set of tools that let you skip the drudgery and get to the creative parts right away. Godot [1] is one of the most popular free and open source game engines, and, after a couple of weeks playing with it, I can see why.
The Concept
One of Godot's creators, Juan "reduz" Linietsky, stated that the name "Godot" is a reference to the homonymous gentleman in Samuel Beckett's play. Godot, in the play, never arrives and, in a similar manner, Linietsky says the Godot game engine will never be entirely finished, as it can always be improved and expanded.
After six years of active development, Godot has grown to include a huge variety of tools. The best way to demonstrate Godot's capabilities is simply to build a game from beginning to end. So let's make a game of tactical interstellar warfare that … Who am I kidding? It's Space Invaders; we're making Space Invaders, people (Figure 1).
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
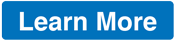
News
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.
-
KaOS 2025.05 Officially Qt5 Free
If you're a fan of independent Linux distributions, the team behind KaOS is proud to announce the latest iteration that includes kernel 6.14 and KDE's Plasma 6.3.5.
-
Linux Kernel 6.15 Now Available
The latest Linux kernel is now available with several new features/improvements and the usual bug fixes.
-
Microsoft Makes Surprising WSL Announcement
In a move that might surprise some users, Microsoft has made Windows Subsystem for Linux open source.