Secure communication over the unreliable UDP transport with DTLS
Secret Delivery
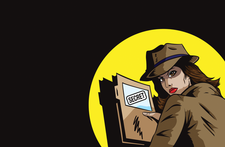
© Lead Image © Daniel Villeneuve, 123RF.com
TLS encryption is wonderful if it is running over a reliable transport protocol like TCP; but if your needs call for the less reliable UDP transport, you'd better start learning about DTLS.
TCP/IP is at the heart of the Internet, and the Transport layer is at the heart of TCP/IP. The Transport layer is responsible for end-to-end connections between the sender and receiver over a TCP/IP network. The two most common Transport layer protocols are Transmission Control Protocol (TCP) and User Datagram Protocol (UDP). Nearly all Internet traffic uses either TCP or UDP.
TCP is connection-oriented, which means that a connection between the client and server is established before data can be sent. The TCP protocol provides reliable ordering and error-checked delivery. UDP is a connectionless protocol, which means it provides only minimal information and has no handshaking procedure. UDP does not offer a guarantee of ordering or delivery. Of course, the brevity of UDP makes it much faster than the steady and careful TCP, so applications that don't require a high level of reliability tend to use UDP.
TCP and UDP were created in more innocent days of an Internet, when networks did not face the security challenges we deal with today. The Transport Layer Security (TLS) protocol (and its predecessor SSL) were developed to provide encryption for communication security at the Transport layer. TLS offers privacy and data integrity between two communicating network nodes; however, it requires a reliable transport protocol, which means it won't work with the simple and unreliable UDP. TLS assumes that the packets arrive in the correct order, which TCP ensures but UDP does not.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
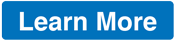
News
-
EU Sovereign Tech Fund Gains Traction
OpenForum Europe recently released a report regarding a sovereign tech fund with backing from several significant entities.
-
FreeBSD Promises a Full Desktop Installer
FreeBSD has lacked an option to include a full desktop environment during installation.
-
Linux Hits an Important Milestone
If you pay attention to the news in the Linux-sphere, you've probably heard that the open source operating system recently crashed through a ceiling no one thought possible.
-
Plasma Bigscreen Returns
A developer discovered that the Plasma Bigscreen feature had been sitting untouched, so he decided to do something about it.
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.