An introduction to electronic weighing
In this article, I discuss the design of a compact and portable workshop balance for various single-load-cell weighing applications constructed with a small load cell, an instrumentation amplifier, an excitation supply, a microcontroller, a display, and a serial port for debugging. Throughout, I used Linux and open source software, and I provide code samples, with directions for finding the complete code online.
History
Ancient civilizations used simple balances to compare weights for trading in precious metals, spices, salt, and the like. Today's civilization is no less dependent on knowing the weight of objects. It is hard to imagine a day in which the knowledge of weight does not take part: from the morning visit to the bathroom scales, a trip to the supermarket, baking a cake, to weighing baggage at the airport – the list of times weight plays a part in our lives seems endless. Today, we've moved away from mechanical balances, for the most part, obviating the need for ready reference weights.
Today's weighing equipment is usually based on electronic signals from strain gauges. These sensors are thin-film resistors whose resistance varies in response to tension or compression. When bonded to a mechanical structure subject to the force of an applied mass, the resistance of a strain gauge will change proportionally in response. Practical weighing systems use more than one strain gauge, and these are generally bonded to a metallic billet in a controlled manner to form a more complex electrical circuit designed to eliminate nonlinearities and temperature effects. These billets are known as load cells and are available commercially with working ranges from a few grams to hundreds of tonnes.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
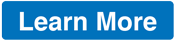
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.