Cross-platform game and app development for new programmers
Dynamic Screens
Ren'Py supports custom screen layouts that can have labels, text, buttons, and bar charts. In a visual novel, a custom screen could be a small box at the top of the application that shows items like inventory, money, or percent complete.
For the next example, I use Python code with Ren'Py to find some PC hardware readings and present the information on a large custom screen.
The Linux sensors
[5] command can be used to show current hardware information:
$ sensors dell_smm-virtual-0 Adapter: Virtual device Processor Fan: 2721 RPM CPU: +46.0 C Ambient: +39.0 C ...
By adding some Bash/Awk code, the CPU and ambient temperature values can be extracted:
# use show Bash/Awk code to get temps $ sensors | grep CPU | awk '{ printf "%d\n" , $2}' 46 $ sensors | grep Ambient | awk '{ printf "%d\n" , $2}' 39
Python calls Bash scripts with the subprocess.check_output
method, which is part of the subprocess library (Listing 2).
Listing 2
subprocess.check_output
$ python Python 2.7.15+ (default, Oct 7 2019, 17:39:04) [GCC 7.4.0] on linux2 >>> import subprocess >>> subprocess.check_output("sensors | grep CPU | awk '{ printf \"%d\" , $2}'", shell=True) '46' >>> >>> subprocess.check_output("sensors | grep Ambient | awk '{ printf \"%d\" , $2}'", shell=True) '39'
Listing 3 shows the code required for the CPU statistics application (Figure 8). The screen cpu_data():
statement creates a user interface (line 3) that can contain both Python blocks and display elements.
Listing 3
Dynamic CPU Stats Screen
01 # script.rpy - create a Ren'Py screen that shows CPU sensor information 02 03 screen cpu_data(): 04 05 python: # Use Python to get sensor variables 06 now = datetime.now() 07 nowtime = now.strftime("%H:%M:%S") 08 ctemp = subprocess.check_output("sensors | grep CPU | awk '{ printf \"%d\" , $2}'", shell=True) 09 atemp = subprocess.check_output("sensors | grep Ambient | awk '{ printf \"%d\" , $2}'", shell=True) 10 11 frame: # create a frame with text, values and a bar 12 has vbox 13 label "CPU Stats" text_size 120 14 15 vbox: 16 text "Time : [nowtime]" size 80 17 text "Ambient temp: [atemp] C \n" size 80 18 text " CPU temp : [ctemp] C " size 60 19 hbox: 20 vbox: 21 text "0 " size 40 22 vbox: 23 bar value atemp range 60 xalign 50 yalign 50 xmaximum 600 ymaximum 50 left_bar "#FF0000" 24 vbox: 25 text " 60 " size 40 26 27 init python: 28 # Define Libaries and any system variables 29 import subprocess 30 from datetime import datetime 31 32 label start: 33 34 # Start with Weather screen 35 show screen cpu_data() 36 37 define cycle = "True" 38 # Cycle every 2 seconds 39 while cycle == "True" : 40 $ renpy.pause(2) 41 42 return
The Python block (lines 5-9), start with a python:
statement, and the succeeding lines are indented per the Python standard. CPU and ambient temperature variables (lines 8-9) are created with the subprocess.check_output()
call and the earlier Bash sensors
statements.
For this screen, a frame:
(line 11) is used to group the elements together. Vertical boxes (vbox:
) and horizontal boxes (hbox:
) are used for further groupings. Python variables are inserted into text output strings with square brackets (lines 16-18). A horizontal bar (line 23) shows the ambient temperature (atemp
) with a range of 0-60.
The application begins at label start:
(line 32) by showing the cpu_data
screen (line 35). An inline Python statement (line 40) pauses the execution for two seconds within a while
loop. The screen logic is refreshed after each renpy.pause()
iteration.
Building Applications
The Ren'Py IDE has a Launch Project button that allows testing in the native operating system environment. The IDE's Build option allows you to create a variety of different packages (Figure 9).
The HTML5 build is still in beta; it appears to work well for standard visual novel apps, but I found that it had issues with some Python library calls. The HTML5 build creates a separate directory structure for the Ren'Py application that needs to be mapped into your web server configuration.
If you are looking to do some simple testing, a Python standalone web server (Figure 10) can be run from the project's web directory:
# Run a python standalone server on port 8042 python3 -m http.server 8042
Final Thoughts
If you're looking to create some simple cross-platform visual presentation apps, Ren'Py is a good fit, it is super easy to learn, and doesn't require strong programming skills. I found the first-time call-up for Android was longer than expected, but I was impressed with the final result.
Ren'Py supports simple screens that can have dynamic bars and text; however, if you're looking to incorporate gauges or line charts. Ren'Py probably isn't the best fit.
Infos
- Ren'Py: https://www.renpy.org
- Download Ren'Py: https://www.renpy.org/latest.html
- Character Creator: https://charactercreator.org
- Bruce Peninsula, Ontario: https://visitbrucepeninsula.ca
- lm_sensors (sensors) documentation: https://wiki.archlinux.org/title/lm_sensors
« Previous 1 2
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
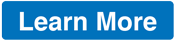
News
-
Gnome 47.1 Released with a Few Fixes
The latest release of the Gnome desktop is all about fixing a few nagging issues and not about bringing new features into the mix.
-
System76 Unveils an Ampere-Powered Thelio Desktop
If you're looking for a new desktop system for developing autonomous driving and software-defined vehicle solutions. System76 has you covered.
-
VirtualBox 7.1.4 Includes Initial Support for Linux kernel 6.12
The latest version of VirtualBox has arrived and it not only adds initial support for kernel 6.12 but another feature that will make using the virtual machine tool much easier.
-
New Slimbook EVO with Raw AMD Ryzen Power
If you're looking for serious power in a 14" ultrabook that is powered by Linux, Slimbook has just the thing for you.
-
The Gnome Foundation Struggling to Stay Afloat
The foundation behind the Gnome desktop environment is having to go through some serious belt-tightening due to continued financial problems.
-
Thousands of Linux Servers Infected with Stealth Malware Since 2021
Perfctl is capable of remaining undetected, which makes it dangerous and hard to mitigate.
-
Halcyon Creates Anti-Ransomware Protection for Linux
As more Linux systems are targeted by ransomware, Halcyon is stepping up its protection.
-
Valve and Arch Linux Announce Collaboration
Valve and Arch have come together for two projects that will have a serious impact on the Linux distribution.
-
Hacker Successfully Runs Linux on a CPU from the Early ‘70s
From the office of "Look what I can do," Dmitry Grinberg was able to get Linux running on a processor that was created in 1971.
-
OSI and LPI Form Strategic Alliance
With a goal of strengthening Linux and open source communities, this new alliance aims to nurture the growth of more highly skilled professionals.