Advanced Bash techniques for automation, optimization, and security
Shell scripting is an essential skill for IT professionals working in Linux environments. Although many users are familiar with the basics of scripting, mastering advanced techniques can elevate your ability to automate tasks, optimize workflows, and manage complex systems efficiently. This article goes beyond scripting fundamentals to explore how advanced shell scripting can solve real-world challenges in Linux-based infrastructures.
Advanced shell scripting requires technical skill and also an adherence to best practices that ensure your scripts are robust, maintainable, and efficient. As scripts grow in complexity, structuring them thoughtfully and implementing techniques like error handling and debugging becomes essential. By following these practices, IT professionals can create scripts that are reliable and adaptable, especially in dynamic Linux environments where automation is key to productivity.
Readability and Maintainability
The foundation of a good script lies in its structure. A well-organized script is easier to understand, debug, and extend. Start by clearly separating different sections of the script, such as initialization, variable declarations, functions, and the main execution block. Use comments generously to explain the purpose of each section and any non-obvious logic. For instance, a comment before a function to describe its input, output, and role in the script makes it much easier for others (and future you) to comprehend.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
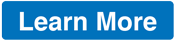
News
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.