Open source messaging middleware
RabbitMQ Messaging
There are a variety of ways to which AMQP messages can be published and subscribed to (Figure 4). The simplest way is to create a queue, and then messages can be published and subscribed to from that queue.
To help with the distribution and filtering of messages, AMQP supports a number of different exchange types. Messages in an exchange use bindings based on a routing key to link them to a queue.
The main types of exchanges are direct, fanout, headers, and topic. An IoT example of a direct exchange would be if a group of Raspberry Pi sensor values were going into a Rasp Pi sensor exchange. When the Rasp Pi publishes a sensor result to the exchange, the message also includes a routing key to link the message to the correct queue (Figure 5).
An IoT example of a fanout exchange would be critical sensor failures. A sensor failure message is sent to Bill and Sam's work queues and the All Maintenance Points queue all at the same time (Figure 6).
Connecting MQTT
After the MQTT plugin is installed, RabbitMQ can act as a standalone MQTT broker.
For an MQTT project, any ESP8266-supported Arduino hardware can be used. There are a number of MQTT Arduino libraries that are available. For this project, I used the PubSubClient library that can be installed using the Arduino Library Manager.
As a test project, I used a low cost MQ-2 Smoke Gas Sensor ($3) [3] that measures a combination of LPG, alcohol, propane, hydrogen, CO, and even methane. Note to fully use this sensor, some calibration is required. On the MQ-2 sensor, the analog signal is connected to Arduino pin A0 and the analogRead(thePin)
function is used to read the sensor value.
Listing 2 is an Arduino example that reads the MQ-2 sensor and publishes the result to the RabbitMQ MQTT broker with a topic name of mq2_mqtt
.
Listing 2
arduino_2_mqtt.ino
01 #include <ESP8266WiFi.h> 02 #include <PubSubClient.h> 03 04 // Update these with values suitable for your network. 05 const char* ssid = "your_ssid"; 06 const char* password = "your_password"; 07 const char* mqtt_server = "192.168.0.121"; 08 const char* mqtt_user = "admin1"; 09 const char* mqtt_pass= "admin1"; 10 11 const int mq2pin = A0; //the MQ2 analog input pin 12 13 WiFiClient espClient; 14 PubSubClient client(espClient); 15 16 void setup_wifi() { 17 // Connecting to a WiFi network 18 WiFi.begin(ssid, password); 19 while (WiFi.status() != WL_CONNECTED) { 20 delay(500); 21 Serial.print("."); 22 } 23 Serial.println("WiFi connected"); 24 Serial.println("IP address: "); 25 Serial.println(WiFi.localIP()); 26 } 27 28 void reconnect() { 29 // Loop until we're reconnected 30 Serial.println("In reconnect..."); 31 while (!client.connected()) { 32 Serial.print("Attempting MQTT connection..."); 33 // Attempt to connect 34 if (client.connect("Arduino_Gas", mqtt_user, mqtt_pass)) { 35 Serial.println("connected"); 36 } else { 37 Serial.print("failed, rc="); 38 Serial.print(client.state()); 39 Serial.println(" try again in 5 seconds"); 40 delay(5000); 41 } 42 } 43 } 44 45 void setup() { 46 Serial.begin(9600); 47 setup_wifi(); 48 client.setServer(mqtt_server, 1883); 49 } 50 51 void loop() { 52 char msg[8]; 53 if (!client.connected()) { 54 reconnect(); 55 } 56 57 sprintf(msg,"%i",analogRead(mq2pin)); 58 client.publish("mq2_mqtt", msg); 59 Serial.print("MQ2 gas value:"); 60 Serial.println(msg); 61 62 delay(5000); 63 }
Once the MQTT value is published, any MQTT client can subscribe to it. Listing 3 is a Python MQTT subscribe example.
Listing 3
mqtt_sub.py
01 import paho.mqtt.client as mqtt 02 03 def on_message(client, userdata, message): 04 print ("Message received: " + message.payload) 05 06 client = mqtt.Client() 07 client.username_pw_set("admin1", password='admin1') 08 client.connect("192.168.0.121", 1883) 09 10 client.on_message = on_message #attach function to callback 11 12 client.subscribe("mq2_mqtt") 13 client.loop_forever() #start the loop
Read MQTT Messages Using AMQP
MQTT clients can subscribe to MQTT messages directly, or it's possible to configure RabbitMQ to have the MQTT data accessible using AMQP. The routing of MQTT messages to AMQP queues is done using the amp.topic direct exchange (Figure 7).
To configure RabbitMQ to forward MQTT, the following steps are required:
- Create a new RabbitMQ queue.
- Create a binding between the MQTT exchange and the queue.
These two steps can be done in a number of ways: manually, in the RabbitMQ config file, using the rabbitmqadmin
command-line tool, or via a program. To use rabbitmqadmin
enter:
./rabbitmqadmin declare queue name=mq2_amqp durable=true ./rabbitmqadmin declare binding source=amq.topic \ destination_type=queue destination=mq2_amqp routing_key=mq2_mqtt
rabbitmqadmin
can also be used to check the queue for messages (Listing 4).
Listing 4
Checking the Queue for Messages
01 ./rabbitmqadmin get queue=mq2_amqp 02 +-------------+-----------+---------------+---------+ 03 | routing_key | exchange | message_count | payload | 04 +-------------+-----------+---------------+---------+ 05 | mq2_mqtt | amq.topic | 77 | 157 | 06 +-------------+-----------+---------------+---------+
« Previous 1 2 3 Next »
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
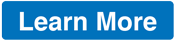
News
-
Canonical Releases Ubuntu 24.04
After a brief pause because of the XZ vulnerability, Ubuntu 24.04 is now available for install.
-
Linux Servers Targeted by Akira Ransomware
A group of bad actors who have already extorted $42 million have their sights set on the Linux platform.
-
TUXEDO Computers Unveils Linux Laptop Featuring AMD Ryzen CPU
This latest release is the first laptop to include the new CPU from Ryzen and Linux preinstalled.
-
XZ Gets the All-Clear
The back door xz vulnerability has been officially reverted for Fedora 40 and versions 38 and 39 were never affected.
-
Canonical Collaborates with Qualcomm on New Venture
This new joint effort is geared toward bringing Ubuntu and Ubuntu Core to Qualcomm-powered devices.
-
Kodi 21.0 Open-Source Entertainment Hub Released
After a year of development, the award-winning Kodi cross-platform, media center software is now available with many new additions and improvements.
-
Linux Usage Increases in Two Key Areas
If market share is your thing, you'll be happy to know that Linux is on the rise in two areas that, if they keep climbing, could have serious meaning for Linux's future.
-
Vulnerability Discovered in xz Libraries
An urgent alert for Fedora 40 has been posted and users should pay attention.
-
Canonical Bumps LTS Support to 12 years
If you're worried that your Ubuntu LTS release won't be supported long enough to last, Canonical has a surprise for you in the form of 12 years of security coverage.
-
Fedora 40 Beta Released Soon
With the official release of Fedora 40 coming in April, it's almost time to download the beta and see what's new.