Solving a classic interview problem with Go
Programming Snapshot – Go Slices
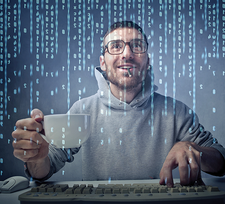
© Lead Image © bowie15, 123rf
Springtime is application time! Mike Schilli, who has experience with job application procedures at companies in Silicon Valley, digs up a question asked at the Google Engineering interview and explains a possible solution in Go.
The TechLead [1], Patrick Shyu, is a YouTube celebrity whose videos I like to watch. The former Google employee, who has also completed a gig at Facebook, talks about his experiences as a software engineer in Silicon Valley in numerous episodes on his channel (Figure 1). His trademark is to hold a cup of coffee in his hand and sip it pleasurably every now and then while he repeatedly emphasizes that he's the "tech lead." That's how Google refers to lead engineers who set the direction for the other engineers on the team. The first-line managers there traditionally stay out of technical matters and focus primarily on staffing and motivating their reports.
One episode on the TechLead channel is about typical questions asked at interviews at Google, of which the former employee says he has conducted hundreds. In this Snapshot issue, we'll tackle one of the quiz questions that he allegedly invented himself and kept asking, a slightly modified version of the flood fill problem [2]. The latter is so well-known that by now any candidate can rattle off the solution blindfolded. That's why Google has removed it from the list of questions, and the TechLead created his own version [3].
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
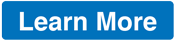
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.