Keep control of goroutines with a Context construct
Go's goroutines are so cheap that programmers like to fire them off by the dozen. But who cleans up the mess at the end of the day? Basically, Go channels lend themselves to communication. A main program may need to keep control of many goroutines running simultaneously, but a message in a channel only ever reaches one recipient. Consequently, the communication partners in this scenario rely on a special case.
If one or more recipients in Go try to read from a channel but block because there is nothing there, then the sender can notify all recipients in one fell swoop by closing the channel. This wakes up all the receivers, and their blocking read functions return with an error value.
This is precisely the procedure commonly used by the main program to stop subroutines that are still running. The main program opens a channel and passes it to each subroutine that it calls; the subroutines in turn attach blocking read functions to it. When the main program closes the channel later on, the blocks are resolved, and the subroutines proceed to do their agreed upon cleanup tasks – usually releasing all resources and exiting.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
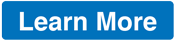
News
-
IBM Announces Powerhouse Linux Server
IBM has unleashed a seriously powerful Linux server with the LinuxONE Emperor 5.
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.