How Perl programmers efficiently manage asynchronous program flows
Even experts can unintentionally build race conditions into their code. Even they can easily overlook the side effects when multithreading suddenly interrupts the flow of code. Emergency teams later have to discover the causes, which involves a huge amount of effort. After all, the error the customer reports occurs only sporadically, because it only occurs given the temporal coincidence of certain events that cannot be easily reproduced by the development department.
One Thread Is Enough
If only one thread is running – as in a JavaScript application – then there can be no such race conditions, because the CPU runs the code as written, and nothing unexpected interrupts the flow. In other words, this approach has its benefits.
For a program with only one thread to run as fast as one with multiple threads, however, it must not be allowed to sit and twiddle its thumbs while, for example, a much slower network operation is in progress. To allow this to happen, the programmer relinquishes control over selected parts of the program to an event loop, which executes the events asynchronously. Once a programmer's brain has mastered the jump to asynchronous program flows, even complicated processes are wonderfully easy to write. Before that happens, however, some hurdles must be taken.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
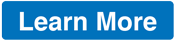
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.