Instant Android Apps
Instant Android Apps
Jasonette makes it supremely easy to build simple and advanced Android apps with a minimum of coding.
For someone who doesn't write code for a living, creating even the simplest Android app can be a daunting proposition. However, for those of us with modest coding skills, there is Jasonette [1]. This ingenious solution makes it possible to create a full-featured Android app using a single JSON file that defines the app's basic structure and behavior. A generic Jasonette Android app pulls this JSON file and renders it as native Android components.
Creating a Jasonette-based app still requires some basic coding skills, but writing a JSON file is significantly easier than writing Java or Kotlin code. Also, building the Jasonette app in the Android Studio IDE requires only a few simple steps. Better still, because the JSON file controls the app's appearance and behavior, you can easily update and improve it by editing the JSON code instead of rebuilding the entire app.
Preparatory Work
Before you start, you need to do some preparatory work. First, download and install Android Studio [2] on your machine. If you happen to use openSUSE, installing Android Studio is a matter of running the following commands:
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
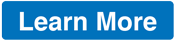
News
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.