Needle in a Haystack
If you have a lot of text files, slide shows, and spreadsheets on your computer, you will need, sooner or later, to know quickly which files contain certain words or sentences. You might also want to use that information to perform some other actions automatically, like sending email notifications or adding new records to a database. Sometimes, you can do this with the Recoll desktop search engine described in the previous issue of Linux Pro Magazine [1]. Should you, however, want something lighter or more flexible than Recoll, try odfgrep
: It not only might work better, but also teach you other, very efficient ways to manage all your office documents.
What and Why
A really basic knowledge of the command line and Bash syntax is helpful, but not mandatory: The code is short and explained as accurately as possible, to help you learn some basics of shell programming, if needed.
In fact, the hardest part of this whole tutorial may not be the code itself, but figuring out why you might want to learn and use it. In a nutshell, learning how to search or otherwise process ODF files from the command line, with odfgrep
or similar tools, can help you to become a much more productive desktop user, able to delegate to your computer many more otherwise very time-consuming tasks. That's it, really.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
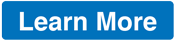
News
-
CachyOS Now Lets Users Choose Their Shell
Imagine getting the opportunity to select which shell you want during the installation of your favorite Linux distribution. That's now a thing.
-
Wayland 1.24 Released with Fixes and New Features
Wayland continues to move forward, while X11 slowly vanishes into the shadows, and the latest release includes plenty of improvements.
-
Bugs Found in sudo
Two critical flaws allow users to gain access to root privileges.
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
LibreOffice Tested as Possible Office 365 Alternative
Another major organization has decided to test the possibility of migrating from Microsoft's Office 365 to LibreOffice.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.