Halloween candy vending machine
With a project as complex as a Halloween vending machine, you need to take everything step by step; otherwise, you quickly get confused. To begin, you must clarify what purpose the vending machine serves. The young visitors are supposed to start it themselves; it then performs a scary show and hands out a piece of chocolate. The show includes sound and light effects – which doesn't sound too complicated – at first.
Chassis
A stable wooden beam forms the basis of the machine. All of the components can be attached to it easily. The temptation to use warped beams just because they are available and cost almost nothing will probably cause more trouble than spending money on good material. The specific machine from this example has a height of around 190cm (~6ft) with a footprint of 60x60cm (~2x2ft). However, no precise size and material specifications are made at this point, just a few tips.
Above all, you need to make sure that the body does not easily tilt or tip over. For this purpose, it is useful to add weights to the base (e.g., heavy stones). Wheels to roll the machine are useful, too. If you construct the vending machine in your garage or basement, test beforehand whether it will fit through the doors that deliver it to its final destination. I have had to disassemble and shorten a completely finished construction in the past just to get it outside.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
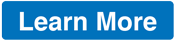
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.