An introduction to electronic weighing
In this article, I discuss the design of a compact and portable workshop balance for various single-load-cell weighing applications constructed with a small load cell, an instrumentation amplifier, an excitation supply, a microcontroller, a display, and a serial port for debugging. Throughout, I used Linux and open source software, and I provide code samples, with directions for finding the complete code online.
History
Ancient civilizations used simple balances to compare weights for trading in precious metals, spices, salt, and the like. Today's civilization is no less dependent on knowing the weight of objects. It is hard to imagine a day in which the knowledge of weight does not take part: from the morning visit to the bathroom scales, a trip to the supermarket, baking a cake, to weighing baggage at the airport – the list of times weight plays a part in our lives seems endless. Today, we've moved away from mechanical balances, for the most part, obviating the need for ready reference weights.
Today's weighing equipment is usually based on electronic signals from strain gauges. These sensors are thin-film resistors whose resistance varies in response to tension or compression. When bonded to a mechanical structure subject to the force of an applied mass, the resistance of a strain gauge will change proportionally in response. Practical weighing systems use more than one strain gauge, and these are generally bonded to a metallic billet in a controlled manner to form a more complex electrical circuit designed to eliminate nonlinearities and temperature effects. These billets are known as load cells and are available commercially with working ranges from a few grams to hundreds of tonnes.
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
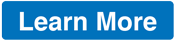
News
-
Plasma Ends LTS Releases
The KDE Plasma development team is doing away with the LTS releases for a good reason.
-
Arch Linux Available for Windows Subsystem for Linux
If you've ever wanted to use a rolling release distribution with WSL, now's your chance.
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.