RFID reader on a Raspberry Pi
Radio-frequency identification (RFID) tags have become indispensable in industry and government, as well as the wholesale and retail spaces. The inexpensive transponder chips can be found on clothing labels, identification cards, and credit cards. Armed with just a Raspberry Pi and an RFID kit, you can read the data from these chips and view it on a display.
In this project, I read serial numbers from RFID tags stuck on 3D-printed pumpkins – a slightly different kind of detection task. To do this, I connect an RC522 [1] RFID kit and a 1.8-inch ST7735 serial peripheral interface (SPI) thin-film transistor (TFT) display [2] to a Raspberry Pi 4. Together, the two modules can cost less than $15 (EUR15, £14) in online stores. The pumpkins contain simple RFID tags [3], also available for very little cash. Although at first glance the project seems clear-cut and sounds as if it should work right away, check out the "Mishaps, Misfortunes, and Breakdowns" box to find out what can go wrong.
The circuit diagram in Figure 1 shows how the modules connect to the Raspberry Pi, along with two pushbuttons and LEDs for testing purposes. The KiCad layout of the project is included in the download section of this article [4].
[...]
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
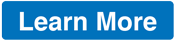
News
-
Fedora Continues 32-Bit Support
In a move that should come as a relief to some portions of the Linux community, Fedora will continue supporting 32-bit architecture.
-
Linux Kernel 6.17 Drops bcachefs
After a clash over some late fixes and disagreements between bcachefs's lead developer and Linus Torvalds, bachefs is out.
-
ONLYOFFICE v9 Embraces AI
Like nearly all office suites on the market (except LibreOffice), ONLYOFFICE has decided to go the AI route.
-
Two Local Privilege Escalation Flaws Discovered in Linux
Qualys researchers have discovered two local privilege escalation vulnerabilities that allow hackers to gain root privileges on major Linux distributions.
-
New TUXEDO InfinityBook Pro Powered by AMD Ryzen AI 300
The TUXEDO InfinityBook Pro 14 Gen10 offers serious power that is ready for your business, development, or entertainment needs.
-
Danish Ministry of Digital Affairs Transitions to Linux
Another major organization has decided to kick Microsoft Windows and Office to the curb in favor of Linux.
-
Linux Mint 20 Reaches EOL
With Linux Mint 20 at its end of life, the time has arrived to upgrade to Linux Mint 22.
-
TuxCare Announces Support for AlmaLinux 9.2
Thanks to TuxCare, AlmaLinux 9.2 (and soon version 9.6) now enjoys years of ongoing patching and compliance.
-
Go-Based Botnet Attacking IoT Devices
Using an SSH credential brute-force attack, the Go-based PumaBot is exploiting IoT devices everywhere.
-
Plasma 6.5 Promises Better Memory Optimization
With the stable Plasma 6.4 on the horizon, KDE has a few new tricks up its sleeve for Plasma 6.5.