Advanced Bash techniques for automation, optimization, and security
Memory and CPU
Monitoring memory and CPU usage is essential for maintaining system stability, especially in environments with high workloads or limited resources. Tools like top
, htop
, and vmstat
provide real-time monitoring, whereas ps
and /proc
offer data for programmatic analysis.
For example, to monitor the memory and CPU usage of a specific process, use ps
:
ps -o pid,comm,%cpu,%mem -p <PID>
This command displays the process ID, the command, and its percentage of CPU and memory usage. In a script, you can automate resource monitoring and trigger alerts if thresholds are exceeded (Listing 7).
Listing 7
Monitoring and Triggering
pid=1234 cpu_usage=$(ps -o %cpu= -p $pid) mem_usage=$(ps -o %mem= -p $pid) if (( $(echo "$cpu_usage > 80" | bc -l) )); then echo "Warning: Process $pid is using $cpu_usage% CPU." fi if (( $(echo "$mem_usage > 70" | bc -l) )); then echo "Warning: Process $pid is using $mem_usage% memory." fi
For long-term monitoring, the sar
utility (part of the sysstat package) records system activity, providing historical data for performance tuning. To view CPU and memory usage trends, use:
sar -u 1 5 # CPU usage sar -r 1 5 # Memory usage
This data can guide decisions on scaling, such as upgrading hardware or distributing workloads across multiple servers.
Testing and Version Control
Ensuring the reliability and maintainability of shell scripts is crucial in production environments, particularly as scripts grow in complexity and integrate with larger systems. Testing and version control play pivotal roles in achieving these objectives. I'll describe how to implement unit testing for shell scripts using tools like bats, integrate shell scripts into CI/CD pipelines for automated testing and deployment, and follow best practices for version control in scripting projects.
Unit Testing
Unit testing is a critical step in verifying the correctness of your shell scripts. The Bash Automated Testing System (bats
) is a lightweight testing framework specifically designed for shell scripts. You can use bats
to write test cases for individual script functions or commands, ensuring they behave as expected under various conditions.
To get started, install bats
on your Linux system. For most distributions, you can install it via a package manager:
sudo apt install bats # Debian-based sudo yum install bats # RHEL-based
Alternatively, you can install bats
using Git:
git clone https://github.com/bats-core/bats-core.git cd bats-core sudo ./install.sh /usr/local
Once bats
is installed, create a test file with the .bats
extension. For example, if you are testing a script called my_script.sh
that calculates the sum of two numbers, your test file might look like the file in Listing 8.
Listing 8
Test File
# test_my_script.bats @test "Addition works correctly" { result=$(./my_script.sh add 2 3) [ "$result" -eq 5 ] } @test "Handles missing arguments" { result=$(./my_script.sh add 2) [ "$result" = "Error: Missing arguments" ] }
Run the tests with:
bats test_my_script.bats
The framework outputs a clear pass/fail summary, making it easy to identify issues. You can extend tests to cover edge cases, invalid inputs, and integration scenarios.
Buy this article as PDF
(incl. VAT)
Buy Linux Magazine
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Subscribe to our ADMIN Newsletters
Support Our Work
Linux Magazine content is made possible with support from readers like you. Please consider contributing when you’ve found an article to be beneficial.
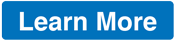
News
-
System76 Releases COSMIC Alpha 7
With scores of bug fixes and a really cool workspaces feature, COSMIC is looking to soon migrate from alpha to beta.
-
OpenMandriva Lx 6.0 Available for Installation
The latest release of OpenMandriva has arrived with a new kernel, an updated Plasma desktop, and a server edition.
-
TrueNAS 25.04 Arrives with Thousands of Changes
One of the most popular Linux-based NAS solutions has rolled out the latest edition, based on Ubuntu 25.04.
-
Fedora 42 Available with Two New Spins
The latest release from the Fedora Project includes the usual updates, a new kernel, an official KDE Plasma spin, and a new System76 spin.
-
So Long, ArcoLinux
The ArcoLinux distribution is the latest Linux distribution to shut down.
-
What Open Source Pros Look for in a Job Role
Learn what professionals in technical and non-technical roles say is most important when seeking a new position.
-
Asahi Linux Runs into Issues with M4 Support
Due to Apple Silicon changes, the Asahi Linux project is at odds with adding support for the M4 chips.
-
Plasma 6.3.4 Now Available
Although not a major release, Plasma 6.3.4 does fix some bugs and offer a subtle change for the Plasma sidebar.
-
Linux Kernel 6.15 First Release Candidate Now Available
Linux Torvalds has announced that the release candidate for the final release of the Linux 6.15 series is now available.
-
Akamai Will Host kernel.org
The organization dedicated to cloud-based solutions has agreed to host kernel.org to deliver long-term stability for the development team.